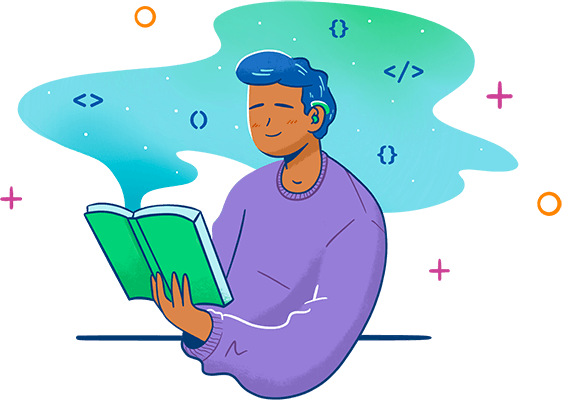
Welcome to the Treehouse Library
This is a sample catalog of all the courses we offer. Browse by topic or difficulty. Sign up today and get access to our entire library. Treehouse students get access to workshops, bonus content, conferences, and more.
Ready to start learning?
Treehouse offers a 7 day free trial for new students. Get access to 1000s of hours of content. Learn to code, land your dream job.
Start Your Free Trial- Newest
-
All Topics
- All Topics
- • AI
- • JavaScript
- • React
- • No-Code
- • Python
- • Design
- • HTML
- • CSS
- • Game Development
- • Data Analysis
- • Development Tools
- • Databases
- • Security
- • Digital Literacy
- • Swift
- • Java
- • Machine Learning
- • APIs
- • Professional Growth
- • Computer Science
- • Ruby
- • Quality Assurance
- • PHP
- • Go Language
- • Android
- • Learning Resources
- • College Credit
- • Coding for Kids
-
Track
Front End Web Development
Learn to code websites using HTML, CSS, and JavaScript.
- Explore
-
An entry-level salary for the technologies covered in this track is about $51,000 / yr on average.
-
Some companies that use these technologies regularly include: Google, Facebook, Yahoo, eBay, Amazon, and Treehouse.
-
Track
Beginning Python
Learn the general purpose programming language Python and build large and small applications and tools.
- Explore
-
An entry-level salary for the technologies covered in this track is about $70,000 / yr on average.
-
Some companies that use these technologies regularly include: Google, NASA, Nokia, IBM, Digg, Dropbox, Pinterest, Reddit, Yelp, Apple, and Amazon.
-
Track
Full Stack JavaScript
Learn JavaScript, Node.js, and Express to become a professional JavaScript developer.
- Explore
-
An entry-level salary for the technologies covered in this track is about $65,000 / yr on average.
-
Some companies that use these technologies regularly include: Walmart, PayPal, Groupon, Airbnb.
Topics
Browse content by the topics that interest you most.
- AI
- JavaScript
- React
- No-Code
- Python
- Design
- HTML
- CSS
- Game Development
- Data Analysis
- Development Tools
- Databases
- Security
- Digital Literacy
- Swift
- Java
- Machine Learning
- APIs
- Professional Growth
- Computer Science
- Ruby
- Quality Assurance
- PHP
- Go Language
- Android
- Learning Resources
- College Credit
- Coding for Kids
Change Your Career, Change Your Life
With 100s of courses and more to come, Treehouse is the best way to learn how to code.
Start Your Free Trial-
- 1
- 2
- 3
- 4
JavaScript Basics
JavaScript is a programming language that drives the web: from front-end user interface design to server-side backend programming, you'll find JavaScript at every stage of a website and web application. In this course, you'll learn the fundamental programming concepts and syntax of the JavaScript programming language.
-
- 1
- 2
- 3
- 4
HTML Basics
Learn HTML (HyperText Markup Language), the language common to every website. HTML describes the basic structure and content of a web page. If you want to build a website or web application, you'll need to know HTML.
-
- 1
- 2
- 3
- 4
Python Basics
Learn the building blocks of the wonderful general purpose programming language Python.
-
- 1
- 3
Python for Kids
Learn the building blocks of the wonderful general purpose programming language Python.
-
- 1
- 2
- 3
- 4
CSS Basics
In this course, we're going to learn the basics of CSS, one of the core technologies for designing and building websites and applications. No matter what kind of website or web application you want to build, you'll have to use CSS. If you haven't written much CSS, or even if you’ve never written CSS at all, don’t worry. That's what this course is for. We’ll start with basic CSS concepts, then gradually progress to more advanced topics and lessons.
-
- 1
- 2
- 3
- 4
CSS Layout
In this course, we're going to learn techniques for better control over our CSS layouts. We’ll cover how the CSS Box Model impacts the presentation of each HTML element, learn to control the position of each element onscreen, and even begin to adjust our layouts for different screen sizes and environments.
-
- 1
- 2
- 3
JavaScript and the DOM
JavaScript lets you create interactive web pages which can respond to a user's actions. In this course, you'll learn how to bring web pages to life using the power of JavaScript.
-
- 1
- 2
- 3
SQL Basics
In SQL Basics, we’ll take a look at what databases are and how you can retrieve information from them. Databases can store massive amounts of information to be retrieved at a later date. Databases act as the memory for dynamic web sites or mobile apps.
-
- 1
- 2
- 3
- 4
Node.js Basics
In this course we will create two command line applications using the popular server-side JavaScript platform Node.js. We'll be creating an application to retrieve a student's Treehouse profile information and be working with a dictionary API to retrieve definitions of a given word.
-
- 1
- 2
- 3
Introduction to Git
Git is a version control system - it helps you manage the different versions of your project files, and helps keep your work safe. This course will show you how Git works, and how to upload your projects to GitHub.
-
- 1
- 2
- 3
- 4
- 5
C# Basics
C# is the most popular programming language in the Microsoft ecosystem of products. C# code is designed to run fast and to be easily maintainable. In C# Basics, we'll learn how to work with C# to write simple programs.
-
- 1
How to Give and Receive Feedback
Learn why feedback is useful. You'll explore a simple process for providing feedback (both positive and constructive) in a way that will be well-received and motivate the right behavior. You'll also learn tips for receiving feedback effectively and putting it into action.
-
9 minWorkshop
JavaScript Search
Letting users search through data in your application or website is a great UX feature. Building out this type of functionality isn't as hard as you may think. With some basic JavaScript, we can tackle the filtering and searching of data. Follow along as I add a search feature with vanilla JavaScript that allows the user to search for a specific author in a list of authors!
Viewed -
2 minWorkshop
Shorten Text With CSS
Ever wondered how to shorten text with an ellipsis (...)? It’s quite easy to do and only requires a few lines of CSS. Follow along as I take you through setting this up!
Viewed -
3 minWorkshop
HTML-Only Accordion
Accordions are all over the web and mobile apps. They are a great way to show and hide content based on user interaction and aren’t very hard to build. However, they usually require a bit of HTML, CSS, and JavaScript. Did you know you can build a simple accordion with only HTML in seconds? Follow along as I explain how to set this up!
Viewed -
8 minWorkshop
Full Stack JavaScript Techdegree Portfolio Overview
Here at Treehouse, we have many Techdegrees to get you job ready no matter your skill level. The Full Stack JavaScript Techdegree is one of the most popular. Come take a look at all the professional-quality projects you'll build in this Techdegree!
Start your free trial today! (https://trhou.se/3I1UNT3)
Projects Random Quote Generator (https://teamtreehouse.com/library/introducing-the-random-quote-generator-2) Data Pagination and Filtering (https://teamtreehouse.com/library/introducing-the-data-pagination-and-filtering-project-2) Interactive Form (https://teamtreehouse.com/library/introducing-the-interactive-form-project-2) OOP Game Show App (https://teamtreehouse.com/library/introducing-the-oop-game-show-app-2) Public API Requests (https://teamtreehouse.com/library/introducing-the-api-requests-project-2) Static Node.js and Express Site (https://teamtreehouse.com/library/introducing-the-static-nodejs-and-express-site-project-2) React Gallery App (https://teamtreehouse.com/library/introducing-the-react-gallery-app-project-2) SQL Library Manager (https://teamtreehouse.com/library/introducing-the-sql-library-manager-project-2) REST API (https://teamtreehouse.com/library/introducing-the-rest-api-project-2) Full Stack App with React and a REST API (https://teamtreehouse.com/library/introducing-the-full-stack-app-with-react-and-a-rest-api-project-2)Viewed
-
- 1
Practice Classes in JavaScript
Practice building and working with classes in JavaScript.
-
11 minPractice
Practice Setting Up a Python Project
Practice setting up a Python project locally and on GitHub.
Viewed -
11 minPractice
Practice Hooks in React
Practice React's built-in useContext and useState Hooks to update an app with user authentication.
Viewed
-
12 hoursConference
Treehouse Festival December 2020
Treehouse Festival is an online conference designed for Treehouse students and all aspiring developers and designers. The presentations will empower those that attend with skills and a network to transition to a tech career or level-up in their current roles. Sessions include “Designing with a Developer Mindset,” “Computer Science, Emulation, and the NES,” “Interview with a Treehouse Grad”, and more!
Viewed -
14 hoursConference
Treehouse Festival June 2021
Treehouse Festival is an online conference designed for Treehouse students and all aspiring developers and designers. The presentations will empower those that attend with skills and a network to transition to a tech career or level-up in their current roles. Sessions include “Designing with a Developer Mindset,” “Computer Science, Emulation, and the NES,” “Interview with a Treehouse Grad”, and more!
Viewed -
12 hoursConference
Treehouse Festival July 2020
Treehouse Festival 2020
Viewed
Bonus Series
Bonus material is exclusive to Courses Plus membership and includes series covering new processes in design, development and illustration.
-
9 minBonus Series
Takeaways
Got some time during your lunch break? Want to get something to takeaway?
Viewed -
3 hoursBonus Series
Treehouse Live
Enjoy our full collection of Treehouse Live sessions with our amazing Treehouse Instructors! Follow along as they teach live and take questions from students like you.
Viewed -
6 hoursBonus Series
Treehouse Guest Speaker Series
Treehouse Guest Speaker Series is an ongoing live event hosted by Treehouse staff. In this series experts working in the field offer advice on how to transition into tech. Want advice on how to nail a technical interview? Need to stand out to tech recruiters? Have the desire to improve your practical skills? This is the series for you!
Viewed -
21 minBonus Series
How to Learn
Learning can be challenging. But having the proper mindset and a good set of strategies for studying and practice can help you learn faster and better. In this series of videos learn helpful techniques to boost your learning, and discover what the latest scientific research has to tell us about how to learn.
Viewed
Upcoming Releases
The following items are scheduled to be released soon. You can also visit our content roadmap for more info.
-
3 minWorkshop
Hello Swift & SwiftUI
Learn the basics of Swift and SwiftUI, Apple’s programming language, by building your first iOS app! This beginner-friendly course covers Swift programming, Xcode setup, and UI design with SwiftUI. You'll apply your skills by creating a Dice Roller app, using state to update the UI dynamically. No prior coding experience needed—just dive in and start building!
Viewed -
Practice Prototyping in Figma
Enhance your prototyping skills by linking mockups in Figma and experimenting with tap, time, and drag interactions.
Viewed -
Unity Basics
Begin your journey into Game Development with the Unity game engine. Unity is a powerful cross-platform game engine that supports both 2D and 3D graphics. This course will guide you through installing Unity and its essential tools, where you'll use C#, the primary programming language used in Unity, to bring your game ideas to life. We'll take a hands-on approach, building your first game that will introduce you to core game development concepts!
-
Java Arrays (2025 Refresh)
Arrays are a container object that allow you store many values of the same type in a single variable