Full Stack JavaScript
Full stack JavaScript developers are highly in-demand. They know how to work with the frontend, backend, and server side of a web application—the "full stack"—via JavaScript.
JavaScript powers the interactivity and user experience on every major website. From user interfaces that provide richer desktop-like experiences to real-time data and messaging applications, you'll find JavaScript in the browser, on your phone, and on the server.
Full stack JavaScript developers working with different frameworks, libraries and languages will know how to use JavaScript in nearly every facet of their work.
In this track, we'll introduce you to the JavaScript language, and the ins-and-outs of adding interactivity in the browser.
Once you're up to speed with JavaScript in the browser, you'll learn Node.js, a popular JavaScript platform for writing and using JavaScript applications outside of the browser. For example, command line applications for improving your workflow, dynamic websites, and much more.
Start your Full Stack Developer journey with this Track!
-
An entry-level salary for the technologies covered in this track is about $65,000 / yr on average.
-
Some companies that use these technologies regularly include: Walmart, PayPal, Groupon, Airbnb
Ready to start learning?
Treehouse offers a 7 day free trial for new students. Get access to 1000s of hours of content. Learn to code, land your dream job.
Start Your Free Trial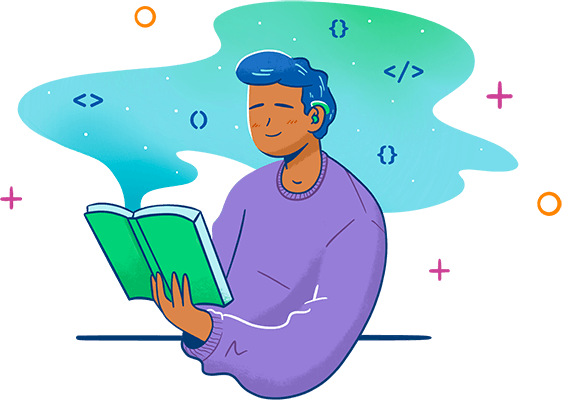
-
- 1
- 2
- 3
- 4
JavaScript Basics
JavaScript is a programming language that drives the web: from front-end user interface design to server-side backend programming, you'll find JavaScript at every stage of a website and web application. In this course, you'll learn the fundamental programming concepts and syntax of the JavaScript programming language.
-
- 1
- 2
JavaScript Numbers
Numbers are everywhere in programming. You use them to track a player's score in a game, calculate the cost of shipping a product, or count the number of times a "Like" button gets clicked on a page. In this course, you'll learn how to use numbers for useful tasks in your JavaScript programs, including doing math, converting strings to numbers, generating random numbers, and more.
-
- 1
- 2
- 3
JavaScript Functions
JavaScript functions let you create reusable chunks of code. They make programming faster, easier, and less error-prone. They are also one of the essential concepts in JavaScript programming.
-
- 1
- 2
JavaScript Loops
Loops are a way of repeating code -- they're handy for repetitive tasks. Loops are frequently used for actions that need to run a particular number of times or until a certain condition is true.
-
- 1
- 2
- 3
JavaScript Arrays
Arrays provide a way to store multiple pieces of information. An array is a list of values: numbers, strings, boolean values, or even other arrays. In this course, you'll learn the basics of using arrays as data structures.
-
- 1
- 2
JavaScript Objects
Objects are an essential part of JavaScript; they provide a flexible way to keep track of data by associating a name with a particular value. In this course, you'll learn the basics of JavaScript objects as a data structure (using objects to store key/value pairs).
-
- 1
- 2
The Landscape of JavaScript
JavaScript is everywhere and used in all phases of development from software to hardware. This course walks you through the modern landscape of JavaScript, and what it means to learn and program with JavaScript now and beyond.
-
- 1
- 2
- 3
JavaScript and the DOM
JavaScript lets you create interactive web pages which can respond to a user's actions. In this course, you'll learn how to bring web pages to life using the power of JavaScript.
-
- 1
- 2
Interacting with the DOM
Along with selecting DOM elements in JavaScript we can also write code that gives elements behavior. In this course we will first explore how to watch for interaction and respond using the method addEventListener. We'll learn about different types of events and how we can use the relationships between elements to write more powerful code.
-
- 1
- 2
AJAX Basics
AJAX is an important front-end web technology that lets JavaScript communicate with a web server. It lets you load new content without leaving the current page, creating a better, faster experience for your website's visitors. In this course, you'll learn how AJAX works and how you can use JavaScript to communicate with a web server. We'll use plain JavaScript to create AJAX requests and use the response to dynamically update your web pages. Along the way, you'll build mini-projects to reinforce your learning.
-
- 1
- 2
- 3
- 4
Asynchronous Programming with JavaScript
In this course, you will learn why asynchronous code matters and how to write code that avoids blocking behavior using three approaches: callbacks, promises, and async/await.
-
44 minWorkshop
Working with the Fetch API
Learn how to use the Fetch API to fetch resources.
Viewed -
- 1
- 2
- 3
- 4
Object-Oriented JavaScript
In this course, you'll learn the basics of object-oriented programming in JavaScript along with the new ES2015 Class syntax.
-
- 1
- 2
- 3
- 4
- 5
Object-Oriented JavaScript: Challenge
Practice your object-oriented JavaScript skills by building a fun and interactive 'Four in a Row' game.
-
25 minWorkshop
Exploring JavaScript Conditionals
In this workshop, we'll explore alternatives to if..then statements for controlling program flow. Even if you don't end up using them, you will see these forms appear in other code bases, and it's helpful to know how they work.
Viewed -
- 1
- 2
- 3
- 4
Build a Simple Dynamic Site with Node.js
Node.js is a versatile platform for building all sorts of applications. In this course, we're going to make a dynamic website that displays a Treehouse student's profile information by creating a server that will dynamically generate content, handle URLs, read from files and build a simple template engine.
-
14 minWorkshop
Using npm as a Task Runner
There are other popular JavaScript task runners out there, like Grunt and gulp but you may not need it.
Viewed -
- 1
- 2
- 3
- 4
- 5
- 6
Express Basics
Learn how to use Express.js to build dynamic websites on the Node.js platform. In the process, you'll learn some fundamental HTTP concepts that you can apply to any other framework!
-
22 minWorkshop
Understanding Closures in JavaScript
In this workshop learn how closures are used to preserve data between function calls.
Viewed -
18 minWorkshop
Debug Node Applications with Visual Studio Code
Debugging Node.js is not limited to using console.log. You can use more powerful and efficient tools like the built-in debugger within the Visual Studio Code editor.
Viewed -
- 1
Asynchronous Code in Express
In this course, we’ll look more closely at three approaches to handling asynchronous operations in Express: callbacks, promises, and async/await.
-
- 1
- 2
- 3
- 4
REST APIs with Express
Learn the basics of building out a REST API with Express, a popular framework written for Node.js.
-
- 1
- 2
- 3
SQL Basics
In SQL Basics, we’ll take a look at what databases are and how you can retrieve information from them. Databases can store massive amounts of information to be retrieved at a later date. Databases act as the memory for dynamic web sites or mobile apps.
-
- 1
- 2
- 3
- 4
Modifying Data with SQL
At the heart of a dynamic application is a database. Whether the application is an eCommerce, sports team, social network, or productivity app on your phone, the data needs to change over time. In this course, we'll take a look at the underpinning SQL statements that are needed for every dynamic application.
-
- 1
- 2
- 3
Using SQL ORMs with Node.js
In this course, you'll learn how to use the Sequelize ORM to leverage the power of SQL within your Node.js applications.
-
37 minWorkshop
Using Sequelize ORM With Express
In this workshop, you will use the Sequelize ORM and Express to build a simple CRUD application that's connected to a SQL database.
Viewed -
Track Completion
This course includes:
- JavaScript Basics 3 hours
- JavaScript Numbers 83 min
- JavaScript Functions 2 hours
- JavaScript Loops 76 min
- JavaScript Arrays 2 hours
- JavaScript Objects 67 min
- The Landscape of JavaScript 45 min
- JavaScript and the DOM 2 hours
- Interacting with the DOM 66 min
- AJAX Basics 2 hours
- Asynchronous Programming with JavaScript 2 hours
- Working with the Fetch API 44 min
- Object-Oriented JavaScript 111 min
- Object-Oriented JavaScript: Challenge 2 hours
- Exploring JavaScript Conditionals 25 min
- Build a Simple Dynamic Site with Node.js 2 hours
- Using npm as a Task Runner 14 min
- Express Basics 4 hours
- Understanding Closures in JavaScript 22 min
- Debug Node Applications with Visual Studio Code 18 min
- Asynchronous Code in Express 24 min
- REST APIs with Express 101 min
- SQL Basics 2 hours
- Modifying Data with SQL 79 min
- Using SQL ORMs with Node.js 100 min
- Using Sequelize ORM With Express 37 min