Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial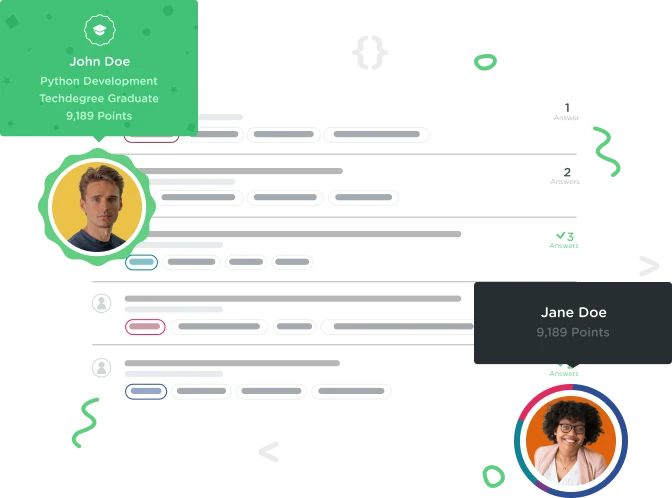

gael blanchemain
Full Stack JavaScript Techdegree Student 8,843 Points"your conditional statement is returning the wrong student level"
I am trying to pass the "getter" test: https://teamtreehouse.com/library/objectoriented-javascript-2/getters-and-setters/creating-getter-methods
My code passes with node js, but not in the treehouse compiler, any hint anyone? Thank you! Here is my code
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
let gpa = this.stringGPA()
if (gpa > 90) {
return 'Senior'
}else if (gpa >= 61){
return 'Junior'
}else if (gpa >= 31){
return 'Sophomore'
} else if (gpa <= 30){
return 'Freshman'
}
}
};
const student = new Student(3.9);
console.log(student.level)
2 Answers
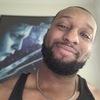
Brandon White
Full Stack JavaScript Techdegree Graduate 35,772 PointsHi gael,
I think the issue you’re having is that you’re comparing the wrong data. The challenge asks that you determine the levels based off of this.credits (not this.stringGPA).
Assuming that the interpreter will compare strings and numbers (because I believe the challenges are already in strict mode), any GPA will always be so small as a number that it will always return Freshman.
Now, it IS odd that in the student variable that they create below the class definition that they haven’t passed in an argument for credits. And maybe that threw you off and is the reason you used the stringGPA as the data you’re testing, but ignore what’s been written on the line below the class definition. Just focus on the instructions and write your code based off that.

gael blanchemain
Full Stack JavaScript Techdegree Student 8,843 PointsI confused myself! your answer was clear, thank you again Brandon White !
gael blanchemain
Full Stack JavaScript Techdegree Student 8,843 Pointsgael blanchemain
Full Stack JavaScript Techdegree Student 8,843 PointsThanks a lot, Brandon!
gael blanchemain
Full Stack JavaScript Techdegree Student 8,843 Pointsgael blanchemain
Full Stack JavaScript Techdegree Student 8,843 PointsI applied your recommendations, but it seems not to run this time again, if you could enlighten me, I would be really grateful!
Brandon White
Full Stack JavaScript Techdegree Graduate 35,772 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,772 PointsIt's possible that I've confused you, gael because you're still not checking this.credits when trying to return the level.
Look over the code I've added, and when you feel like you understand where you were a little off, complete this challenge and keep moving forward.