Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial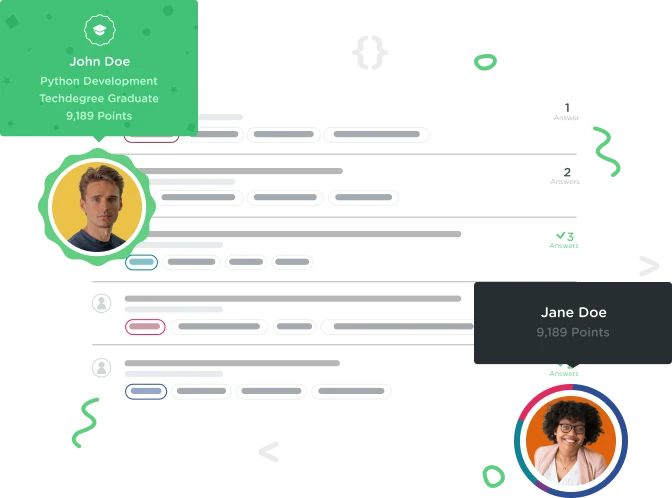
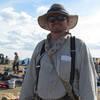
Rune Larsen
25,877 PointsWrong equals Right? Right?
For some reason it don't seems to be right. But I got it right on the challenge tasks.
I feel there is something wrong, as I don't use the IgnoreCase.
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample == secondExample);
console.printf("first is equal to second");
if (firstExample == thirdExample);
console.printf("first is equal to second");
Someone with some light on this shed, please...
5 Answers
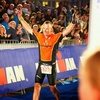
Steve Hunter
57,712 PointsThe comparison between first and third is meant to use the equalsIgnoreCase()
method:
if (firstExample.equalsIgnoreCase(thirdExample)){
console.printf("first is equal to third");
}
The whole challenge would be completed like this:
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample.equals(secondExample)){
console.printf("first is equal to second");
}
if (firstExample.equalsIgnoreCase(thirdExample)){
console.printf("first is equal to third");
}
Some of your output strings were identical - I think the victim of some copy/pasting! ;-)
From way back, I recall being told never to compare equality of strings with ==
but to use the methods equals()
etc. I don't recall why, though it is just something I was taught!
Steve.
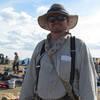
Rune Larsen
25,877 PointsGrigorij,
The reason I feel it is wrong, is that the first and third should only be equal when the equalsIgnoreCase is used. But it did accept the code.
Thanks for modifying my code.
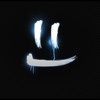
Grigorij Schleifer
10,365 PointsNo problem :)
Glad I could help a little bit
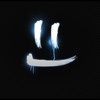
Grigorij Schleifer
10,365 PointsHi Rune,
I have modified your code.
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample == secondExample){
console.printf("first is equal to second");
}
if (firstExample == thirdExample){
console.printf("first is equal to second");
}
You can use equals method too:
if (firstExample.equals(secondExample)){
console.printf("first is equal to second");
}
if (firstExample.equals(thirdExample)){
console.printf("first is equal to second");
}
Grigorij
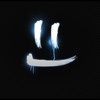
Grigorij Schleifer
10,365 PointsThe "coding kitten"
:))))
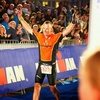
Steve Hunter
57,712 PointsThere's an interesting part of this video where the ==
operator is overridden to work "correctly" - it is done by the IDE in this instance. It is worth a look around the 5m40s mark.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsStack Overflow came to my aid ... here's why not to use
==
to compare strings.Steve.
Rune Larsen
25,877 PointsRune Larsen
25,877 PointsIn this case, it gave the wrong answer a pass. and that started the whole issue. The good thing is that it helped me understand what not to do. And if that causes the challenge to be tighten in, or someone like me comes behind and does the same mistake and don't learn from it, then so be it. Thanks to all who gave input.
I had a kitten playing on my laptop, so I had to do it quickly, and distracted, so I saw later that I had not corrected my copy/paste error.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI agree - the compiler in the online challeges will pass things sometimes if the tests being run aren't broad enough to catch every outcome. I've seen the string comparators being used wrongly before in this way.
Glad you're OK with it - and I hope the kitten behaves! :-)
Steve.