Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial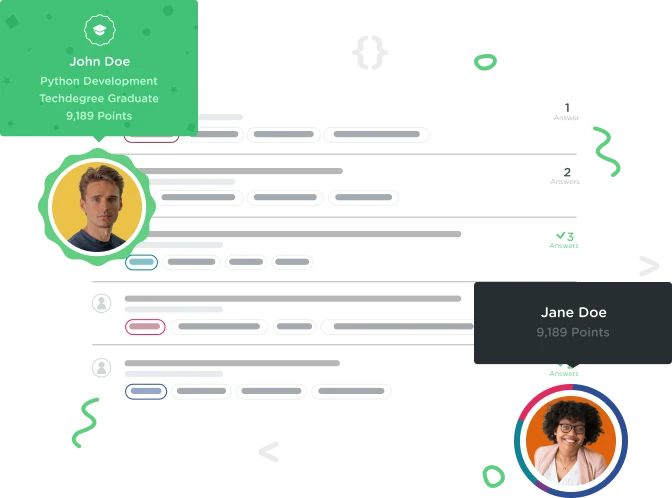
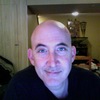
Charles Harpke
33,986 PointsWrite a function named minutes that takes two datetimes and returns the number of minutes, rounded, between them.
Stuck as to how to round the minutes...Here is my code:
import datetime
datetime1 = datetime.datetime.now()
datetime2 = datetime.datetime.now()
def minutes(datetime1, datetime2):
return datetime1 - datetime2
import datetime
datetime1 = datetime.datetime.now()
datetime2 = now.timestamp()
def minutes(datetime1, datetime2):
return datetime1 - datetime2
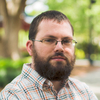
Kenneth Love
Treehouse Guest TeacherPretty similar to what we did in one of the videos, actually.
>>> dt1 = datetime.datetime(2014, 12, 6, 9)
>>> dt2 = datetime.datetime(2014, 12, 6, 10, 30)
So dt1
is a datetime
pointing to today at 9am. dt2
is the same but pointing at 10:30am instead.
>>> td = dt2 - dt1
Since dt1
is older than dt2
, if I want the difference between them, I need to subtract dt1
from dt2
. Just like I'd get the difference between 2 and 10 by doing 10 - 2
instead of 2 - 10
.
If I look at td
, I get:
>>> td
datetime.timedelta(0, 5400)
That's a timedelta
, or difference between two times, that is 0 days and 5400 seconds.
OK, how do I convert seconds to minutes? Well, how many seconds are in a minute? 60! So I'd divide my seconds by 60 to get the number of minutes.
>>> td.seconds / 60
90.0
But the challenge wants a whole number, or integer, for the answer, so we have to use round()
. I'm sure you can do the rest of this.
7 Answers
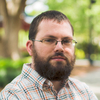
Kenneth Love
Treehouse Guest Teachertimedelta
objects have a few almost-always-present attributes, like we go over in the video. They have days
, seconds
, and microseconds
(there are a couple of others possible but pretty uncommon). So, you have a timedelta
and it'll have seconds
, so how would you use that to find minutes?
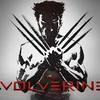
Wolverine .py
2,513 Pointsimport datetime
time_1 = datetime.datetime.now()
time_2 = datetime.datetime.now().replace(minute=59)
def minutes(time_1, time_2):
delta = time_2 - time_1
delta_seconds = delta.total_seconds()
return round(delta_seconds/60)
this worked for me:)

Don Han
7,811 PointsHere's my answer that went through.
import datetime
def minutes(old, new): diff = new - old return round(diff.total_seconds() / 60)
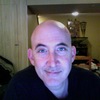
Charles Harpke
33,986 PointsGot it...thank you.

Christos Peramatzis
16,428 Pointsi can't understand why this isn't working
def minutes(date1, date2):
sec1 = date1.total_seconds()
sec2 = date2.total_seconds()
mins = (sec2 - sec1) / 60
return round(mins)
sorry for all the spaces but I could not format it differently
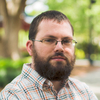
Kenneth Love
Treehouse Guest Teacherdatetime
s don't have total_seconds
, only timedelta
s do. The function receives two datetime
objects. So to get from a datetime
to a timedelta
, what do you need to do?

Christos Peramatzis
16,428 Pointssubtract them..

Trust Mujaka
1,821 Pointsimport datetime def minutes(datetime1, datetime2): return round((datetime2-datetime1).total_seconds()/60)
Charles Harpke
33,986 PointsCharles Harpke
33,986 Pointssorry....still not quite seeing it...