Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial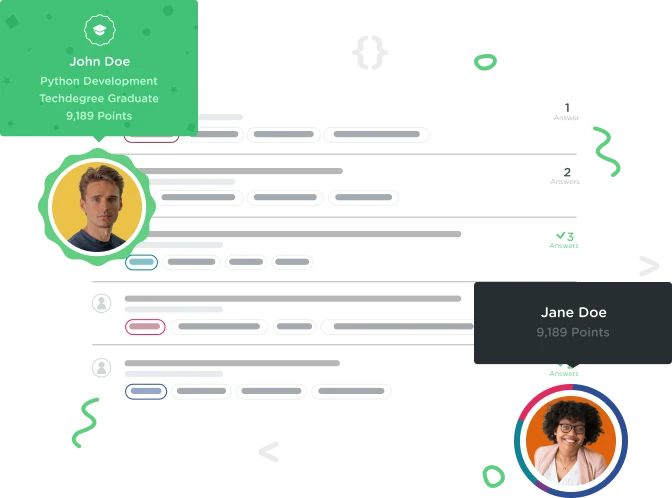
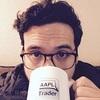
Nicholas Gaerlan
9,501 PointsWorks one way but not the other
for some reason this code works the way I have it shown, but if I write
def __str__(self):
return "-".join(["dot" if x == "." else "dash" if x == "_" for x in self.pattern])
it won't work... why is that?
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
return "-".join(["dot" if x == "." else "dash" for x in self.pattern])
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
3 Answers

Susannah Klanecek
5,825 Pointsdef __str__(self):
output = []
for entry in self.pattern:
if entry == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)

Susannah Klanecek
5,825 PointsActually I think the list comprehension is ok on second thought, seems pretty readable, and the thing you tried didn't work because of indentation under the def function. Still no idea why your list comprehension didn't work though

David Luo
1,215 PointsI did the same thing and it didn't work, but created a temporary variable, say morse, and it worked. I.e., keep the list comprehension separate line from the "".join(morse) part. try that...
def __str__(self):
morse = ["dot" if x == "." else "dash" for x in self.pattern]
return ("-".join(morse))

David Luo
1,215 Pointsnevermind, misread your question. I looked up list comprehension and it doesn't support the syntax as you have it on the first section.
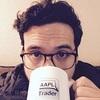
Nicholas Gaerlan
9,501 Pointssince researching this, I've come to a guess that comprehensions can't handle the else-if syntax. Everything you pass into a comprehension basically has to resolve in a binary sort of way. In plain language. "this or that" not "this or that or that or that". there's probaby a way around this creating some kind of other variable that abstracts the string in some way before passing it to a list comprehension, but at that point you are creating a solution to fit the tool instead using a tool to find a solution.
Nicholas Gaerlan
9,501 PointsNicholas Gaerlan
9,501 Pointsactually, this way works fine
and it's more efficient and 'pythonic' using a list comprehension. in your version you also don't bother testing for " _ " which assumes that the only characters in the list would be either "." or " _ ", I was just curious why it wouldn't work if you tested both. Afterall, what if there's a list with more than just "." or " _ " in it? in both of our solutions anything != "." would evaluate to "dash" even if that element was say... a number or a boolean or any other string character.
Nicholas Gaerlan
9,501 PointsNicholas Gaerlan
9,501 PointsYou make a good point on readability. I think it's readable but maybe most would not see comprehensions as "natural" like me. Second point tho... something is not working on this quiz because if I adjust your solution with an elif then it won't work, so there's something wrong and it's not our answers (I think)
that won't work for some reason.