Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial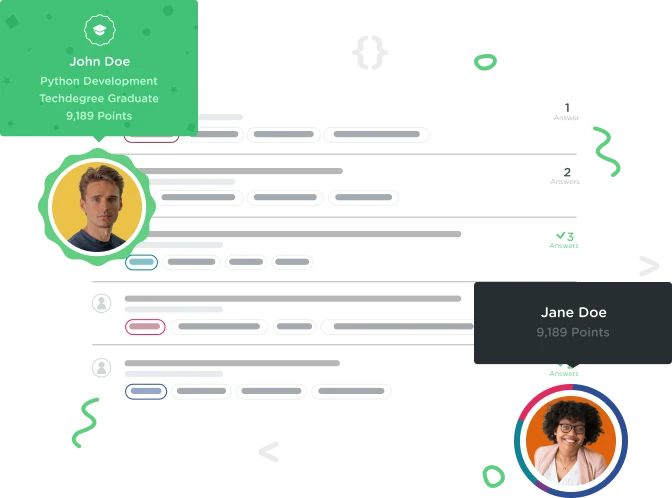
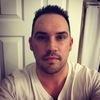
Jesse Reinhalter
1,034 PointsWorking with Switch Statements: Objective 1
I do not know how to append that value to the proper capital array. I've gone ahead and used Switch statements to add the key to the proper array. Should that not infer that the value should now be a part of that array as well?
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key {
case "BEL":
print(europeanCapitals)
case "LIE":
print(europeanCapitals)
case "BGR":
print(europeanCapitals)
case "USA":
print(otherCapitals)
case "MEX":
print(otherCapitals)
case "BRA":
print(otherCapitals)
case "IND":
print(asianCapitals)
case "VNM":
print(asianCapitals)
default:
print(otherCapitals)
}
// End code
}
1 Answer

Chris White
2,308 PointsIt can't infer what you're trying to do from what you've written because you're giving it very different directions.
What you have is basically telling Swift that when it loops through the keys each key is going print the contents of the array you've specified into the console.
Let's simplify this by redefining the first case as a single if then
conditional representing the first true part of the case statement and an else
signifying the default
portion.
for (key, value) in world {
// Enter your code below
if key == "BEL" {
print(europeanCapitals)
} else {
print(otherCapitals)
}
// End code
}
This will return []
in your playground result for the if
part and then seven repetitions of []
for else. In the console you should get the following:
[]
[]
[]
[]
[]
[]
[]
[]
Other than it knowing the variables we're printing is an array it doesn't know what should be in them. Put another way, what we're doing is reading the arrays eight times when we want to be adding information to them.
You may also be getting an error that value
is a mutable type that isn't being used in your expression, this should be a giveaway that something isn't correct.
Instead, we want to add each of those values to the arrays, we can do this two ways. First, since the arrays exist we can use the append
method that is automatically part of the array type:
europeanCapitals.append(value)
We're taking each value and appending it to the array through the dot notation syntax.
The other way we could do it is to concatenate the existing arrays with new ones we're creating each loop through:
europeanCapitals = europeanCapitals + [value]
You can do whichever makes the most sense to you, they do the same thing but the dot notation feels cleaner to me so it's what I originally used when doing this exercise.
Just don't use both, it's best to be consistent or it makes it more difficult for us to read:
switch key {
case "BEL", "LIE", "BGR":
europeanCapitals = europeanCapitals + [value]
case "IND", "VNM":
asianCapitals.append(value)
default:
otherCapitals.append(value)
}
One other thing you might note is that you don't have to explicitly provide the case statements for the otherCapitals
, just set the default and let it catch everything that doesn't fit the other two arrays we've defined.
In real life, I'd probably try to have an appropriate array for each of the values I know I'll be using — in this case that would be an americanCapitals
array (or northAmericanCapitals
and southAmericanCapitals
), only use otherCapitals
as a backup, but given that we're explicitly naming it other I think using the default is more appropriate in the challenge.
Jesse Reinhalter
1,034 PointsJesse Reinhalter
1,034 PointsChris, First, I cannot thank you enough for taking the time to answer my question so thoroughly. You really fleshed out the details and made the work digestible for me. From what I gather, it's safe to say that europeanCapitals = europeanCapitals + [value] is the same as europeanCapitals.append(value)
Chris White
2,308 PointsChris White
2,308 PointsNo problem Jesse, I'm glad I was able to help!
Yes, while they may technically being doing it in a different way they accomplish the same result. I don't know without doing some digging but it's possible the append method for Array type is using the concatenation syntax behind the scenes.
If that doesn't really make much sense yet you'll learn more about how the mechanics of this could work in the Object Oriented Swift class you will working on soon!