Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial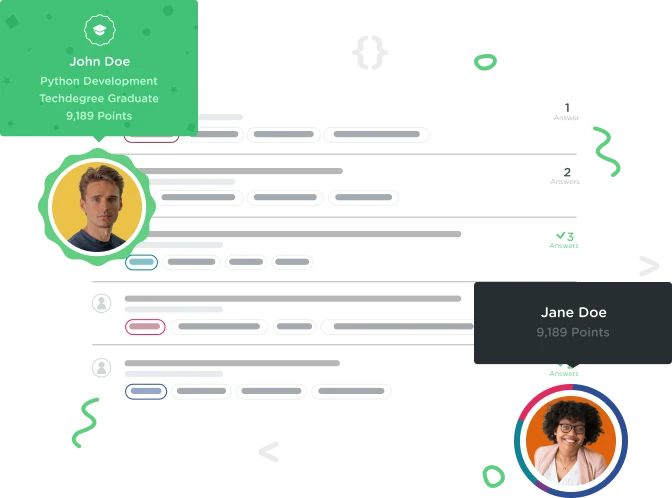

Dean Kreseski
2,418 PointsWorking on building this vending machine app and its giving me this error and I can't figure out why.
func vend(_ quantity: Int, selection: VendingSelection) throws {
guard inventory[selection] != nil else {
throw VendingMachineError.invalidSelection
}
guard Item.quantity >= quantity else {
throw VendingMachineError.outofStock
}
let totalPrice = Item.price * Double(quantity)
if amountDepostied >= totalPrice {
amountDepostied -= totalPrice
Item.quantity -= quantity
inventory.updateValue(Item() as! VendingItem, forKey: selection)
}
}
func deposit(_ amount: Double) {
}
}
The error it keeps giving me is "Instance member "quantity/price" cannot be used on type item" I have already declared a struct named Item that uses the Vending Item protocol and has two properties = price and quantity. Cant seem to find the problem. Any help would be appreciated and if you need more code let me know.

Dean Kreseski
2,418 Pointsstruct Item: VendingItem {
let price: Double
var quantity: Int
}
Let me know if you need any other code, could it be something in the project settings as far as the version of Swift I am using
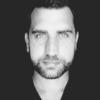
Jhoan Arango
14,575 PointsYou are trying to configure/change a property directly on a type. The "quantity" property is an instance property, not a type property.
Difference between them:
// Instance property
var quantity: Double?
// To use it you must create an instance of the type it belongs to
// Instance of Item
var item = Item(price: 2.0, quantity: 1)
item.quantity -= 1
// type property
static var quantity: Double?
Item.quantity -= 3
See the difference ?
Look into that and you will find your mistake there.
1 Answer
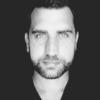
Jhoan Arango
14,575 PointsTry something like this:
func vend(_ quantity: Int, selection: VendingSelection) throws {
// Here we create an instance of "Item" if one exist, if it does not exist
// then the guard will call the error
// if It does exist then the guard will create the instance of item and the execution continues
guard var item = inventory[selection] else {
throw VendingMachineError.invalidSelection
}
guard item.quantity >= quantity else {
throw VendingMachineError.outofStock
}
let totalPrice = item.price * Double(quantity)
if amountDepostied >= totalPrice {
amountDepostied -= totalPrice
item.quantity -= quantity
inventory.updateValue(item, forKey: selection)
}
}
func deposit(_ amount: Double) {
}
}

Dean Kreseski
2,418 PointsSo on the instance property you give values to the properties of the struct and on the type property you just declare the type with or without an optional before you give a value for it? And why is it a instance property and not a type property because its a variable and not a let constant?
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsHello,
Would you share your Item struct ?
From I can see you are trying to modify the "quantity" from a type, meaning that there might not be an instance of said item.