Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial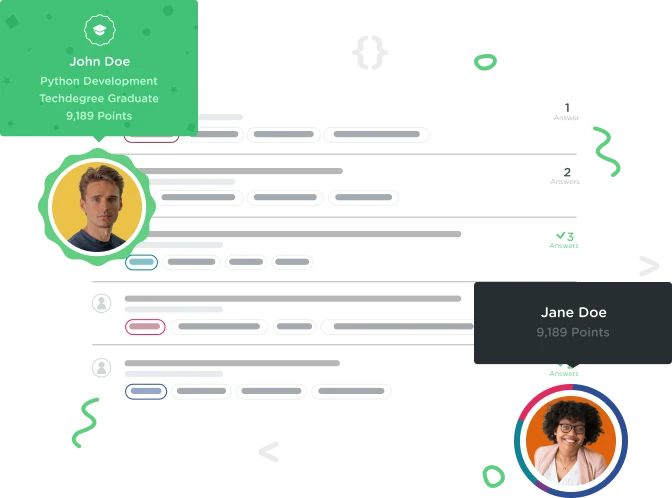
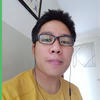
Rifqi Fahmi
23,164 PointsWhy we need to use return keyword in router.get() callback ?
In this project we can see the file at /routes/index.js
var express = require('express');
var router = express.Router();
// GET /
router.get('/', function(req, res, next) {
return res.render('index', { title: 'Home' });
});
// GET /about
router.get('/about', function(req, res, next) {
return res.render('about', { title: 'About' });
});
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});
module.exports = router;
as we can see each callback has "return" keyword. I try removing the word return and it work fine for me. What is the difference between using return and not using return ??
Dave McFarland Thanks :)
1 Answer
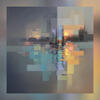
Montazar Al-Jaber
19,611 PointsThere is a subtle difference. When you use the return statement, you are canceling any following middleware. Otherwise, they'll be invoked.
Montazar Al-Jaber
19,611 PointsMontazar Al-Jaber
19,611 PointsCorrection, this is not the case. None of the following middleware will be canceled. JavaScript automatically puts a return statement for you if you omit it. The teacher perhaps puts it for the sake of clarity.
Source: A short guide to Connect Middleware.. I miss interpreted 'all subsequent middleware will be skipped...' as canceling. What the author actually meant is that the error is not handled by any subsequent middleware until it reaches an error handler.
Note: Original entry left for the sake of clarity, in case anyone interprets the article in the same way.
Rifqi Fahmi
23,164 PointsRifqi Fahmi
23,164 PointsMuntazir Al-Jaber I think the following middleware will be canceled. I have done a simple test with code like this :
The console will log the "first middleware" mean that it was executed, then the next middleware is the get() method which display the word "index page" to the browser and the lastMiddleware didn't get executed. Console
first middleware
If I put the return keyword in the firstMiddleware function like this
The console will execute the function and return the value then the next() function will never be executed.
Montazar Al-Jaber
19,611 PointsMontazar Al-Jaber
19,611 PointsRifqi Fahmi, the order in which you use your middleware is important.
Since lastMiddleware is used after a route handler, the server will write and end the response after it. In other words, the call stack will never reach lastMiddleware. This is true regardless of whether you use a return statement or not.
'While not always a pitfall, it is important to understand that even though app.use() is invoked for every HTTP verb*, middleware is processed in order with route handling. ' - Express.js Middleware Demystified.
To illustrate my point. I will simply change the order of the middleware and our route handler.
This time, both of the middleware will log a message.
*An HTTP verb refer to the methods by which a request is sent, like a GET or POST verb. While the resource is referred to as a noun.