Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial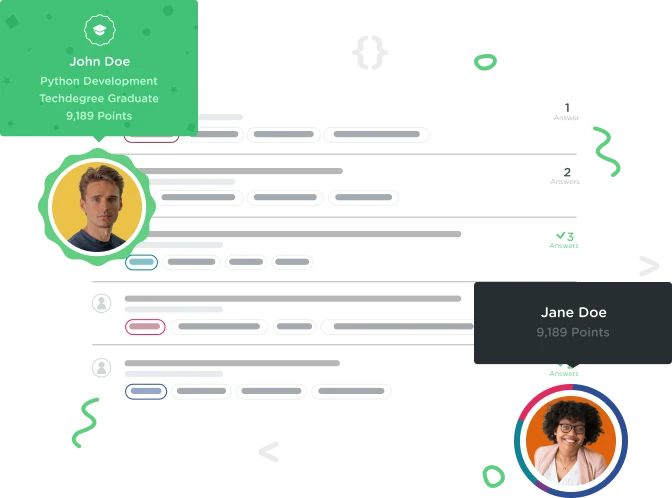
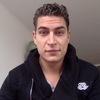
Berry Loeffen
4,303 PointsWhy not use a function?
When working on this question i immediately started making a function.
I thought that you always create a function to do a very specific task. Which matches that criteria in this question.
I am just wondering why this would not be the best idea to use a function.
3 Answers

Thomas Dobson
7,511 PointsIt really depends on the context and use case. Stick to DRY (Dont Repeat Yourself). There is nothing wrong with writing such a task into a function. It also gives you the ability to reuse the code easily if the need arises later.
This was my func in Formatting Time:
func convertSeconds (toHoursMinutesSeconds secondsIn: Int)
{
let hours = secondsIn/3600
let timeMinusHours = secondsIn % 3600
let minutes = timeMinusHours/60
let timeMinusMinutes = timeMinusHours%60
let seconds = timeMinusMinutes
let timebreakdown = "\(secondsIn) seconds converts to \(hours) hours, \(minutes) minutes and \(seconds) seconds. "
print(timebreakdown)
}
I also built a function for the reverse:
func convertHoursMinutesSeconds(toHours: Int, minutes: Int, seconds: Int)
{
let convertSeconds = (toHours*3600)+(minutes*60)+seconds
print("\(toHours) hours, \(minutes) minutes, \(seconds) seconds converts to \(convertSeconds) seconds")
}
these could be useful functions in another set of code one day too. You never know!
I hope this helps.
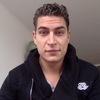
Berry Loeffen
4,303 PointsThanks! just like I thought.

Daniel Walkowski
549 PointsHere is a version that doesn't use %, by subtracting away the the largest to smallest units:
```func stringTime (seconds: Int) -> String {
let second = 1 let minute = 60 * second let hour = 60 * minute
let originalTime = seconds var time = seconds
let hours = time/hour time -= (hours * hour)
let minutes = time/minute time -= (minutes * minute)
let seconds = (time/second) return("(originalTime) seconds is (hours) hours, (minutes) minutes, and (seconds) seconds")
}
///////////////////////////////// print(stringTime(seconds: 50))
print(stringTime(seconds: 69240)) print(stringTime(seconds: 36240))```

Daniel Walkowski
549 PointsDaniel Walkowski 10 minutes ago Here is a version that doesn't use %, by subtracting away the the largest to smallest units:
swift
func stringTime (seconds: Int) -> String {
let second = 1 let minute = 60 * second let hour = 60 * minute
let originalTime = seconds var time = seconds
let hours = time/hour time -= (hours * hour)
let minutes = time/minute time -= (minutes * minute)
let seconds = (time/second) return("(originalTime) seconds is (hours) hours, (minutes) minutes, and (seconds) seconds")
}
print(stringTime(seconds: 69240))
please ignore bad formatting in previous post
Jeff McDivitt
23,970 PointsJeff McDivitt
23,970 PointsWhat question are you referring to?