Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial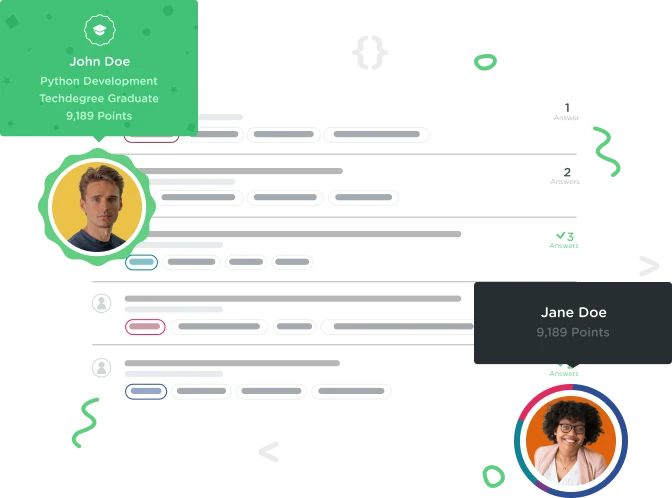
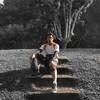
Wan Nor Adzahari Wan Tajuddin
2,438 PointsWhy it doesn't work with System.out.printf ?
My code here won't pass. It is because I used System.out.printf instead of System.out.println. So what I want to know, why it only works with println but not with printf ?
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(42);
} catch (IllegalArgumentException iae) {
System.out.printf("I'm sorry, %s", \\I should have have used System.out.println
iae.getMessage);
}
}
}
class GoKart {
public static final int MAX_BARS = 8;
private int barCount;
private String color;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
lapsDriven += laps;
barCount -= laps;
}
}
2 Answers

andren
28,558 PointsIt is because I used System.out.printf instead of System.out.println.
Actually that is incorrect, it has nothing to do with printf
vs println
. The issue is that you have forgotten to add parenthesis to the iae.getMessage
method. If you add them like this:
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(42);
} catch (IllegalArgumentException iae) {
System.out.printf("I'm sorry, %s",
iae.getMessage());
}
}
}
Then your code will work fine.
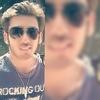
Manas Vijaywargiya
12,598 PointsThe answer is simple- u should use parenthesis after iae.getMessage()
class Example {
public static void main(String[] args) { GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } try { kart.drive(42); } catch (IllegalArgumentException iae) { System.out.printf("I'm sorry, %s", iae.getMessage()); } }
}
Joshua Mclendon
46,708 PointsJoshua Mclendon
46,708 PointsBecause,
System.out.printf("I'm sorry, %s", iae.getMessage);
Should be
System.out.printf("I'm sorry, %s", iae.getMessage());
You forgot the parenthesis in getMessage(). getMessage() is a method that returns your message so methods require () when called by dot notation.