Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial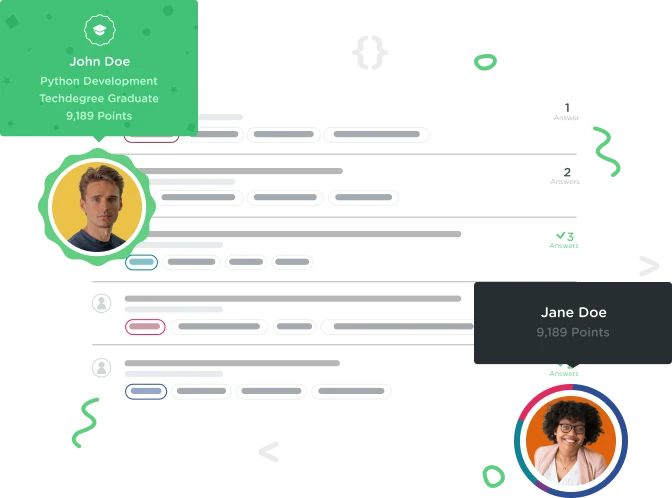
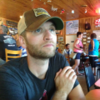
John Barhorst
9,648 PointsWhy is this "for" loop running through my prompt()'s 3 times?
I'm not looking for much more than why my "for" function is popping up my prompt() dialogues nine times instead of just 3.
I'm using the following:
var quizQuestions = [
["How many licks does it take?", "3"],
["Why so serious?", "the batman"],
["Where's the beef?", "wendy's"]
];
var correctAnswers = 0;
function print(message) {
document.write(message);
}
for ( var i = 0; i < quizQuestions.length; i += 1) {
var question1 = prompt(quizQuestions[0][0]);
var question2 = prompt(quizQuestions[1][0]);
var question3 = prompt(quizQuestions[2][0]);
}
I'm just working through how I want to do this quiz challenge yet, so if I'm not even taking the right approach I'd like to figure that out on my own. My issue here is that in trying to test my code the prompts are looping through 3 times for each question, even though using quizQuestions.length in the JavaScript console on Chrome tells me that it is only 3 long.
At first I thought the count might change since I'm using arrays within arrays ( feints within feints! ), but even then wouldn't it end at 6? Shouldn't the counter variable "i" still be adding one each time and ending the 'for' function on the third prompt?
Thanks for reading so far down!
2 Answers

Gilbert Kennen
10,661 PointsYour for loop is set up correctly, it is running its code block 3 times. The problem is that each time it runs it is running 3 prompt() methods. What you want is something that looks more like this:
var quizQuestions = [
["How many licks does it take?", "3"],
["Why so serious?", "The Batman"],
["Where's the beef?", "Wendy's"]
];
for ( var i = 0; i < quizQuestions.length; i += 1 ) {
var answer = prompt(quizQuestions[i][0]);
// code to do something based on the result of answer
}
This for loop runs three times and the value of i will be 0 on the first run, 1 on the second run and 2 on the third run, so it will prompt each of your questions once.
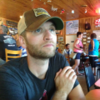
John Barhorst
9,648 PointsAh yeah! That clears it up nicely. Thanks a lot :D
Gilbert Kennen
10,661 PointsGilbert Kennen
10,661 PointsWhen doing code blocks, you can include the name of the language after the opening backticks and you will get the correct syntax highlighting. For example
```JavaScript
// JavaScript code here.
```