Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial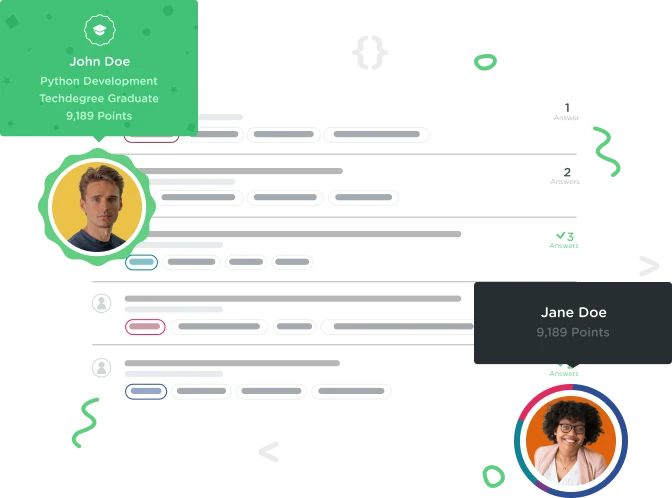

Unsubscribed User
1,653 PointsWhy Is My Program Not Working?
I am trying to figure out how to print "Yay!" on the screen as many times as the user asks but my script is just not working. Can anybody tell me where I slipped up?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
String Awnser = Console.Readline;
int Number = int.Parse(String Awnser);
int Yay = 1
Yay = Console.Write("Yay!");
while (int Yay < int Number);
Yay + 1
}
}
}
1 Answer
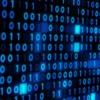
Alexander Davison
65,469 PointsTry this:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
int times = int.Parse(entry);
while (times > 0)
{
Console.WriteLine("Yay!");
times -= 1;
}
}
}
}
Here's a step-by-step explanation of what's going on:
- We are getting the input using the
Console.ReadLine()
function. (Don't forget to use the parentheses when calling a function!) - We convert the input (by default a string and not a number) into a number and store it in a variable called
times
. - We create a
while
loop that runs as long as thetimes
variable is greater than zero. - We print out "Yay!", then decrease
times
by one.
Here's how this works:
"Times" is set to 5 (that's the user input).
C# Prints "Yay!"
"Times" is decreased, now is 4
C# Prints "Yay!"
"Times" is decreased, now is 3
C# Prints "Yay!"
"Times" is decreased, now is 2
C# Prints "Yay!"
"Times" is decreased, now is 1
C# Prints "Yay!"
"Times" is decreased, now is 0
The while loop ends since "Times" is no longer greater than zero.
Good luck! I hope you understand.
~Alex
If you have any questions about the second task, reply below. I'm happy to help :)
Unsubscribed User
1,653 PointsUnsubscribed User
1,653 PointsAmazing. Thanks For That I was really stuck. I will try out task 2 and see if I can manage. Also is that the only way it could be done or is there other ways to complete this task?
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsActually, I believe there's quite a few ways to solve this task :)
For instance, this one is slightly different:
However, I think the first approach is simpler/better.