Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial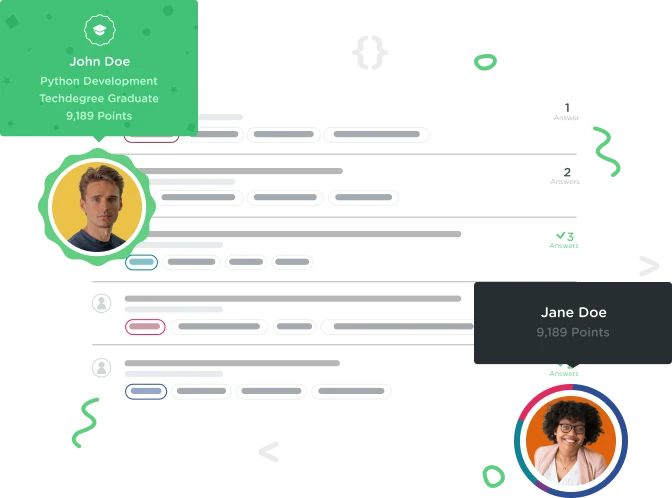
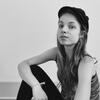
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsWhy is my "for" loop not working properly?
I am trying to create a button element for each corresponding letter in currentQuestion for the quiz I am making. However, when I run the loop. It creates a button element for ALL answers to every question in the quiz. Does anyone know why? Thank you!
var quizQuestionsContainer = document.getElementById("quiz");
var quizQuestion = document.getElementById("quiz-question");
var buttonsContainer = document.getElementById("buttons-container");
var startButton = document.getElementById("start");
startButton.addEventListener("click", function() {
buttonsContainer.removeChild(startButton);
quizQuestions.removeChild
displayQuestion();
});
var displayQuestion = function() {
quizQuestions.forEach ((currentQuestion) => {
currentQuestion.answers
for (letter in currentQuestion.answers) {
var optionButton = document.createElement("button")
optionButton.id = "option-button"
optionButton.textContent = currentQuestion.answers[letter];
buttonsContainer.appendChild(optionButton);
}
quizQuestion.innerText = currentQuestion.question
});
};
// quiz questions
var quizQuestions = [
{
question: "Inside the HTML document, where do you place your JavaScript code?",
answers: {
a: "Inside the <script> element",
b: "Inside the <link> element",
c: "Inside the <head> element",
d: "In the <footer> element"
},
correctAnswer: "a",
feedback: "Nice job! You place your JavaScript inside the <script> element of the HTML Document is correct."
},
{
question: "What operator is used to assign a value to a declared variable?",
answers: {
a: "Double-equal (==)",
b: "Colon (:)",
c: "Equal sign (=)",
d: "Question mark (?)"
},
correctAnswer: "c",
feedback: "Awesome! The correct way to assign a variable is with an equal sign(=)."
},
{
question: "What are the six primitive data types in JavaScript?",
answers: {
a: "sentence, float, data, bigInt, symbol, undefined",
b: "string, number, boolean, bigInt, symbol, undefined",
c: "sentence, int, truthy, bigInt, symbol, undefined",
d: "string, num, falsy, bigInt, symbol, undefined"
},
correctAnswer: "b",
feedback: "Stellar! JavaScript has a total of six primitive data types: string, number, boolean, bigInt, symbol, undefined."
},
{
question: "What is the difference between && and ||?",
answers: {
a: "The logical operator && returns true if both expressions are true while the logical operator || returns true if one expression or the other returns true.",
b: "The logical operator && returns true if one expression is true while the logical operator || returns true if both expressions returntrue true.",
c: "The logical operator && returns true if none of the expressions are true while the logical operator || returns true if one expression or the other returns true.",
d: "The logical operator && returns true if both expressions are true while the logical operator || returns false if one expression or the other returns true."
},
correctAnswer: "a",
feedback: "High-five! The logical operator && returns true if both expressions are true while the logical operator || returns true if one expression or the other returns true. Check out some of the other operators available to you in the MDN Web Docs."
},
{
question: "How do we declare a conditional statement in JavaScript?",
answers: {
a: "difference...between",
b: "for loop",
c: "while loop",
d: "if...else"
},
correctAnswer: "d",
feedback: "Amazing! if... else is most definitely how we declare a conditional statement. This is something you will use every day as a JavaScript developer."
},
{
question: "How do we stop a loop from from repeating indefinitely?",
answers: {
a: "A loop will stop executing when the condition is true.",
b: "We have to explicitly end the loop with the break keyword.",
c: "A loop will stop executing when the condition is false.",
d: "When we have iterated through half of the condition."
},
correctAnswer: "c",
feedback: "Fantastic! In JavaScript a loop will stop executing when the condition is false. Have a look at the documentation to solidify your knowledge of loops."
},
{
question: "Which statement below is not true about functions in JavaScript?",
answers: {
a: "Functions can be reused throughout your code",
b: "A function must always be assigned an identifier",
c: "Functions can receive arguments that can alter the output of a function",
d: "Functions are able to be recursive."
},
correctAnswer: "b",
feedback: "You're doing great! Functions without identifiers are called anonymous functions which are used quite frequently used in JavaScript. Make sure you are familiar with functions and how they work."
},
{
question: "What are the two types of scope JavaScript uses?",
answers: {
a: "Outside and Inside",
b: "Surrounding and Inner",
c: "Abroad and Local",
d: "Global and Local"
},
correctAnswer: "d",
feedback: "Nice job! JavaScript has two forms of scope, global and local. Have a look at the documentation on scope because it is something that will continuously during your JavaScript journey."
},
{
question: "As a developer, I want to be able to remove the last element of my array and I want to also be able to add a new element to the beginning of my array. Which two array methods should I use?",
answers: {
a: "concat() and shift()",
b: "forEach() and pop()",
c: "pop() and unshift()",
d: "push() and sort()"
},
correctAnswer: "c",
feedback: "Awesome! The pop array method removes the last element of an array and the unshift method adds an element to beginning of the array."
},
{
question: "How do we access a value stored in an object?",
answers: {
a: "Period notation, Square bracket notation",
b: "Dot notation, Bracket notation",
c: "Dot notation, Curl bracket notation",
d: "Equal notation, Abstract notation"
},
correctAnswer: "b",
feedback: "Stellar job! We have two ways of accessing data inside of an object, dot notation and bracket notation. Have a look at the documentation to better understand the behavior of objects."
}
];
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Fundamentals Quiz</title>
<link rel="stylesheet" href="./assets/css/style.css">
</head>
<body>
<h1>JavaScript Fundamentals Quiz</h1>
<!-- display questions -->
<div class="quiz-container">
<div id="quiz">
<p id="quiz-question">Quiz Question Text Here</p>
</div>
</div>
<!-- buttons -->
<div id="buttons-container" class="buttons-container">
<button id="start">Start Quiz</button>
</div>
<!-- display results -->
<div id="results"></div>
<script src="./assets/js/script.js"></script>
</body>
</html>
1 Answer
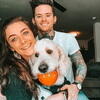
zappbrandigan
21,144 PointsIn the JS file, the quizQuestions.forEach()
is iterating through all of the question in quizQuestions
. One option you could use is to add a questionCounter
and remove the outer iteration. This way buttons will only be added to the current question.
var quizQuestionsContainer = document.getElementById("quiz");
var quizQuestion = document.getElementById("quiz-question");
var buttonsContainer = document.getElementById("buttons-container");
var startButton = document.getElementById("start");
let questionCounter = 0; // added
startButton.addEventListener("click", function() {
buttonsContainer.removeChild(startButton);
quizQuestions.removeChild
displayQuestion();
});
var displayQuestion = function () {
// quizQuestions.forEach ((currentQuestion) => {
//currentQuestion.answers
let currentQuestion = quizQuestions[questionCounter]; // added: use the questionCounter to access the current question
for (letter in currentQuestion.answers) {
var optionButton = document.createElement("button")
optionButton.id = "option-button"
optionButton.textContent = currentQuestion.answers[letter];
buttonsContainer.appendChild(optionButton);
}
quizQuestion.innerText = currentQuestion.question
questionCounter += 1; // added: increment question counter -- this can be handled elsewhere if needed
// });
};
Alternatively, generating the buttons and actually displaying the buttons could be separated into separate functions so that you can control when and which buttons display.
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsKate Johnson
Python Development Techdegree Graduate 20,155 PointsThat is super helpful! Thank you!