Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial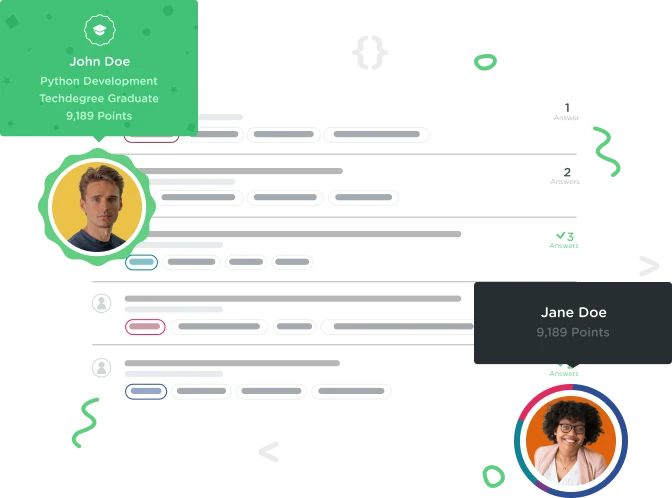

edumorlom
4,073 PointsWhy is it sorting my HashMap automatically. I don't want that.
My code:
import java.util.*;
class Main {
public static void main(String[] args) {
Map<String, Integer> peopleByAge = new HashMap<String, Integer>();
peopleByAge.put("Alvaro", 21);
peopleByAge.put("Eduardo", 19);
peopleByAge.put("Carlos", 13);
peopleByAge.put("Pedro", 29);
System.out.println(peopleByAge);
for (Map.Entry mapEntry : peopleByAge.entrySet()){
System.out.println(mapEntry.getKey() + " is " + mapEntry.getValue() + " years old");
}
}
}
The programs output is:
//Notice how I put Pedro after Carlos, yet HashMap is showing Pedro first.
{Eduardo=19, Alvaro=21, Pedro=29, Carlos=13}
Eduardo is 19 years old
Alvaro is 21 years old
Pedro is 29 years old
Carlos is 13 years old
Also, why is it that when I import java.util.HashMap instead of the entire java.util.* library, my code fails?
1 Answer

markmneimneh
14,132 PointsHello
re:
Why is it sorting my HashMap automatically. I don't want that.
Please read this ... http://beginnersbook.com/2013/12/hashmap-in-java-with-example/
the compiler, by design, does not guarantee that the order you get back when you print out a hashmap, is same as the one you put in.
Hashmap, by definition, is an un-ordered list of key-value items.
re: Also, why is it that when I import java.util.HashMap instead of the entire java.util.* library, my code fails?
probably it is failing because you also need:
import java.util.Map;
I see you are using Map in your example
If this answers the question, please mark the question as answered.
Thanks