Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial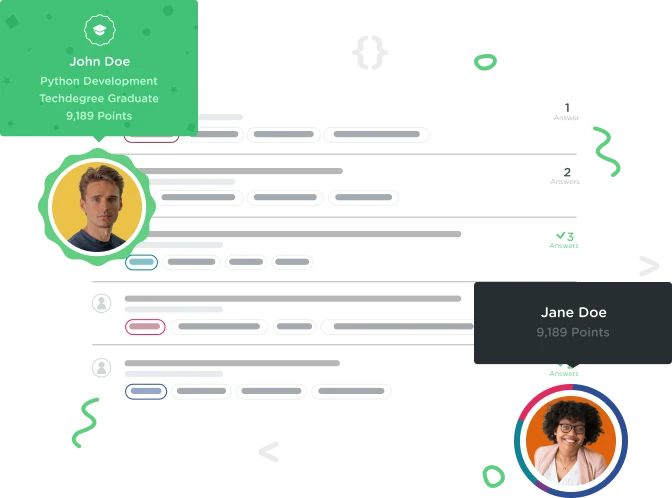

Erik Nilsen
20,433 PointsWhy doesn't this work?
Hey all, I was hoping if someone could tell me why the following fails. I'll first show the "correct" version and then my original one.
// works, and yes, I know there's no error checking
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> videos = course.getVideos();
Video video = videos.get(oldTitle);
video.setTitle(newTitle);
}
// Doesn't work, and I don't know why :(
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> videos = course.getVideos();
Video video = videos.remove(oldTitle); // assigns the value of the old title to the new Video instance
videos.put(newTitle, video); // should put the old video with new title back into the hash
}
My only guess is that my method DOES work, just doesn't maintain order. Can anyone verify this or, if I'm incorrect, tell me what I'm doing wrong? Thanks!
3 Answers
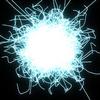
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Eric,
You're super close on this so I'll give a hint. The part of the code that is causing you trouble is
Map<String, Video> videos = course.getVideos();
The video Map isn't properly being imported instead it is the videoList from the couse. Since you got through step Two you already set your Video Map up. All that you need to do is pass your map that you created in the place of this line and leave your two lines below it fine and you should be set.
Video video = videos.get(oldTitle);
video.setTitle(newTitle);
Are exactly right and what you need.
Let me know if this doesn't help and I'll try to further assist.

Thi Dinh La
9,274 PointsHey Erik, may I ask what behavior you are expecting see here? It seems to me that the two functions you posted are doing slightly different things.
The first is taking the video that is associated with the key oldTitle in the videos Map<> and setting its title variable to newTitle.
The second is taking the video that is associated with the key oldTitle in the videos Map<>, and changing the key it is associated with to newTitle within the map. The title member variable of the video is question remains unchanged in this case.

Erik Nilsen
20,433 PointsHi Thi,
I appreciate but don't think I understand your comment. In both examples, I first get the videos, which I assign to the videos
Map. Then, in the first example, I grab a reference to the video with the desired old title and change it to the new one. In the second example, I remove the old title while simultaneously setting a holding variable to the value that was removed and then set a new value in the Map with the same value but different key (ultimately, the correct behavior, in my opinion). I know the approach is different, but I don't understand why the outcome is somehow different.
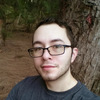
Unsubscribed User
11,389 PointsI was able to keep it to one line with the following:
videosByTitle(course).get(oldTitle).setTitle(newTitle);
videosByTitle(course) gets the Map
get(oldTitle) retrieves the video by its old title
setTitle(newTitle) dynamically changes the title then and there
No need to remove or put and keeps it in place.

Anthony Branze
4,887 PointsThats what if finally took for me to pass 3/3 challenge. Thank you so much I could not figure it out for the life of me
Erik Nilsen
20,433 PointsErik Nilsen
20,433 PointsHi Rob, Thank you for your reply but I'm still a little confused. Both examples I provided, the first of which works, use the
Map<String, Video> videos = course.getVideos();
expression with no modification. The difference only seems to be that in the first example, I get a reference to the video that we want to retitle and change the title in place. In the second example, I completely remove the video but maintain a reference to it, change the title, and reinsert it into the map, thereby likely changing its position in the map. Ultimately though, both methods produce a map with the same contents.Erik Nilsen
20,433 PointsErik Nilsen
20,433 PointsHi Rob, please ignore my previous comment. I think Treehouse may have bugged out because I certainly didn't do it right and it passed me. Neither of my two examples in the original post work and they shouldn't since a map and list are incompatible types. I'm not sure what happened when I was working on this but I got it all resolved now. Thank you for your help!
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Erik,
I'm glad that this worked out for you, this challenge gave me a false positive as well when I first did it for an unrelated reason. I know Craig is working behind these and getting it to work.
Again, glad to hear it got sorted out.