Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial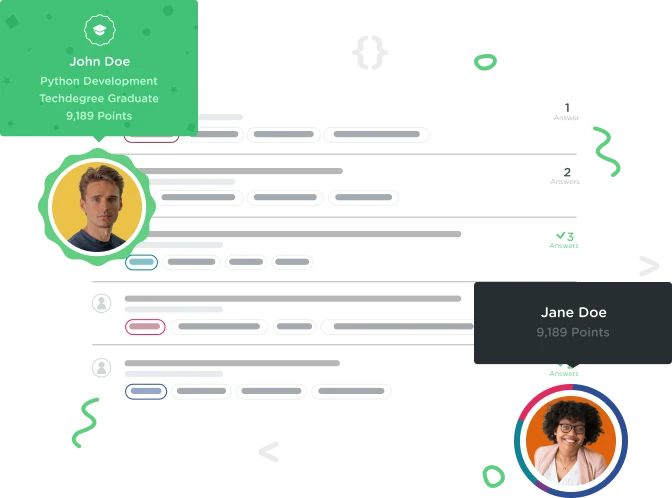

D Anthony
6,045 PointsWhy does this code only need to be ran in the TicTacToe class but not its parent class of Boards?
So I passed this code challenge; however, I feel like I need further explanation as to why this the iter is only needed for the TicTacToe class and not its parent class. I understand making TicTacToe iterable using the iter method, but why would Board not have to be iterable? Or is it already iterable due to the self.cells being a list and if so then why wouldn't its child class also be iterable by default?
Apologies if the wording for this question is confusing, I'm just a little unclear about this particular concept applied to this example.
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self):
super().__init__(width = 3, height = 3)
def __iter__(self):
for item in self.cell:
yield item
3 Answers

andren
28,558 PointsI can't say I fully understand your confusion, as I can't really think of a reason why the parent would have to be iterable. A child class inherits all of the methods and properties from its parent, but aside from that they are entirely separate classes. You can add and change anything you want in a child class without making any changes to the parent class. The parent is essentially just a starting template.
In this challenge the challenge checker will create an instance of the TicTacToe
class and try to iterate through it, when doing so Python will simply look for an __iter__
method in that instance, since it finds that method iterating though the object will work fine. With the above code the Board
class itself would indeed not be iterable, but since there is no code trying to iterate though an instance of Board
that is not an issue.
Can you clarify why you feel that the parent needs to be iterable in the first place? As I mentioned I can't really understand why you assume that to be the case. If you clarify it more then I might be able to give a better explanation.

D Anthony
6,045 PointsHey andren,
Thanks for the response. Believe it or not your answer did suffice. I think I just needed someone to reiterate that to me. I'm not 100% sure why I got confused myself but that clarification makes sense. Often I tend to overthink things even after I've already got code that runs. Thanks for the feedback!

andren
28,558 PointsGlad to hear that. I was not entirely sure my answer would be that useful.
Anyway it's pretty common to be a bit confused about stuff when you first start programming, especially since you will be learning a lot of new stuff all at once. As you start to use the languages more though it usually becomes easier to wrap your mind around exactly what is going on.
Good luck with your continued learning .
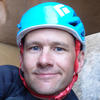
Bob Allan
10,900 PointsNote: the second to last line should read self.cells (not cell) in order for it to work.

andren
28,558 PointsGood catch, I didn't notice that typo since Anthony said the code worked fine. But you are entirely right.