Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial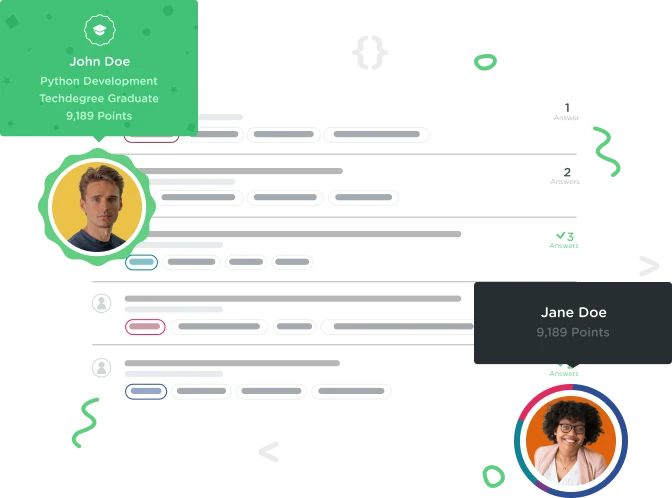

Daniel Escobar
2,580 PointsWhy does my code not work?
'''py def disemvowel(word): result = " " vowels = ["a", "e", "i", "o", "u"]
for letter in word:
word = list(word)
if letter.lower() in word:
continue
else:
result += letter
print(result)
disemvowel("Carlos")
'''
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
for letter in vowels:
if letter.lower('a') == a
letter.replace('a','')
elif letter.lower('e') == 'e':
letter.replace('e','')
elif letter.lower('i') == 'i':
letter.replace('i', '')
elif letter.lower('o') == 'o':
letter.replace('o','')
elif letter.lower('u') == 'u':
letter.replace('u', '')
else:
return word
2 Answers
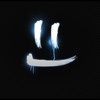
Grigorij Schleifer
10,365 PointsHi Daniel, here my take on your second code. I modified and commented on it. Let me know what you think. One important thing is to use the word argument for the for loop but change the list representation of it. This way you keep checking all characters one by one and if you remove one you don't skip a character. See this answer.
def disemvowel(word):
# include also uppercase letters
# this way you dont need to lower characters
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
word_mutable = list(word)
# you have to iterate over the word and not the vowels
# if you find that letter from the WORD are inside vowels
# delete it in the mutable form of word
# this is because word is a string and strings are immutable
# they cannot be changed
for letter in word:
if letter == "a" or letter == "A":
word_mutable.remove(letter)
elif letter == "e" or letter == "E":
word_mutable.remove(letter)
elif letter == "i" or letter == "I":
word_mutable.remove(letter)
elif letter == "o" or letter == "O":
word_mutable.remove(letter)
elif letter == "u" or letter == "U":
word_mutable.remove(letter)
# no else statement needed
# othewise you would return an unfinished version if no vowel was found
# but you want to remove all vowels first and then return the result
# BUT before you return it you need to convert it back to a sting
# using as sting attribute/function called join()
# join joins the list into a string before return get called
return "".join(word_mutable)
Here also another suggestion that is less verbose:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
word_to_modify = list(word)
for i in word: # loop over the word to prevent index shift and scipping
if i in vowels:
word_to_modify.remove(i)
return "".join(word_to_modify)
Please ask if anything is not entirely clear.

Daniel Escobar
2,580 PointsThis code works for me but not when I try it on the challenge. Can someone tell me why?
'''py
def disemvowel(word):
word = set(list(word))
word_mutable = set(word)
for letter in word:
if letter == "a" or letter == "A":
word_mutable.remove(letter)
elif letter == "e" or letter == "E":
word_mutable.remove(letter)
elif letter == "i" or letter == "I":
word_mutable.remove(letter)
elif letter == "o" or letter == "O":
word_mutable.remove(letter)
elif letter == "u" or letter == "U":
word_mutable.remove(letter)
word_mutable = list(word_mutable)
print(word_mutable)
'''
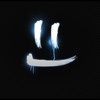
Grigorij Schleifer
10,365 PointsHey Daniel, I will look at your code when I am back home. Sorry for the delay.
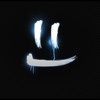
Grigorij Schleifer
10,365 PointsHey Daniel, you are absolutely right. This vowels line can be deleted because all the vowels are caught in the if statements.
Daniel Escobar
2,580 PointsDaniel Escobar
2,580 PointsGrigorij Schleifer, thank you so much, thanks for the answer. So far the best answer. Have a nice and blessed day.
Daniel Escobar
2,580 PointsDaniel Escobar
2,580 Pointsfor the first answer, we technically don't need the vowels list because the if block will match against the WORD right?
''' python
def disemvowel(word):
'''