Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial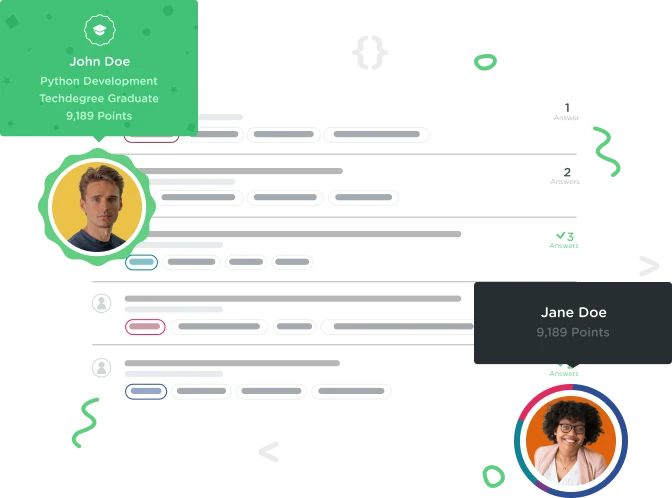
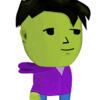
Rafael Moreno
17,642 PointsWhat's the purpose of self in the method
I'm really don't understand what is the purpose of self in the method, I mean how does the self "thing" work ???
I don't understand why we are using it
2 Answers

tangybit
6,777 PointsTo put it simply, a method is another term for a function within a class. There are different types of methods that you can create within a class like class methods, static methods, or instance methods.
For instance methods, you are required to include at least one argument in your method to reference the instance. In almost every case, the term self
is used as the argument. Therefore, you'll know it's an instance method whenever you see this term in Python.
# this is an instance method
def __init__(self, first, last):
self.first = first
self.last = last
To be clear, while self
is the convention, you can really use any word, such as pig or beer. You should, however, follow the the norm to make it easier for others to recognize that it's an instance method.
What happens when you don't include self?
# "first" will replace "self" since it is the first argument
def __init__( first, last):
self.first = first
self.last = last
Whatever your first argument is, it will be used to replaced it. In this case, self
would be replaced by first
. You'll be missing an argument in your method as a result.
Be sure to also precede self
or whatever word you choose in the variable names of your method (e.g. self.first
and self.last
).
Every time you call or run an instance method, a new instance is created:
class Test:
def __init__(self, first, last):
self.first = first
self.last = last
# "student1" is the name of the instance
# "Rafael" and "Moreno" are attributes
student1 = Test('Rafael', 'Moreno')
# this will print your first and last name
print("{} {}".format(student1.first, student1.last))
In this example, student1
is the new instance. Rafael
and Moreno
are the attributes of the instance.
Note that you don't include self
as an argument when you call the method, but it is required when you are first defining the method, otherwise your code will not run, so it can help to think of self
as a place holder.
Main Takeaways:
-
Self
references instances within in method - you can also have class methods and static methods, but every time you seeself
, you know it's an instance method. - Technically, you don't have to use the term
self
. You can use any word you like, but this is the convention. - You absolutely need to include
self
(or any other word) as the FIRST argument in your function or your code will throw an error. In other words, at least one argument is required for your method to run.

Lucas Eiruff
1,446 PointsQuick google search:
The reason you need to use self. is because Python does not use the @ syntax to refer to instance attributes. Python >decided to do methods in a way that makes the instance to which the method belongs be passed automatically, but not >received automatically: the first parameter of methods is the instance the method is called on. That makes methods >entirely the same as functions, and leaves the actual name to use up to you (although self is the convention, and people >will generally frown at you when you use something else.) self is not special to the code, it's just another object.
Python could have done something else to distinguish normal names from attributes -- special syntax like Ruby has, or >requiring declarations like C++ and Java do, or perhaps something yet more different -- but it didn't. Python's all for >making things explicit, making it obvious what's what, and although it doesn't do it entirely everywhere, it does do it for >instance attributes. That's why assigning to an instance attribute needs to know what instance to assign to, and that's >why it needs self..
Anibal Marquina
9,523 PointsAnibal Marquina
9,523 PointsThink of self is the representation of the instances that will create later. As an example lets change "self" for the word "instance".
In this case Rafael is the instance. Instead of using the word 'instance' people normally uses 'self'