Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial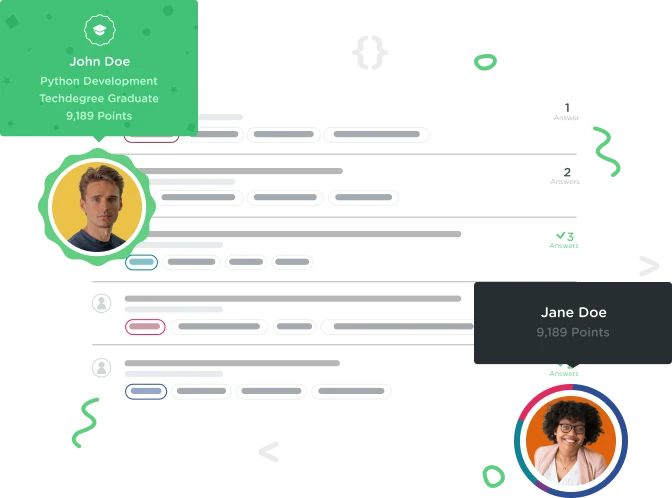

Perfect Storm
1,416 PointsWhat's the difference between append and extend in this example?
I don't understand what differentiates append and extend. Is it that append is for one item as a string and extend is for adding multiple items?
2 Answers
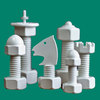
Steven Parker
231,268 PointsThe "append" method adds a single item to a list, though the item might be another list. On the other hand, "extend" will add another list as individual items. You can see this in the example you provided:
list1.append(list2)
list1
['a', 'b', 'c', ['d', 'e', 'f']] # list2 is still a list, INSIDE the big list
list1.extend(list3)
list1
['a', 'b', 'c', ['d', 'e', 'f'], 1, 2, 3] # but 1, 2, and 3 are separate in the big list
Happy coding! And for some holiday-season fun and coding practice, give Advent of Code a try!

nicolaspeterson
8,569 PointsWas just about to ask the same question and found your answer. Many thanks.

aaron r
1,669 Pointsif one can extend a list with single list item, why bother with append?
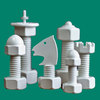
Steven Parker
231,268 PointsYou might want to add something to a list that isn't already in another list.
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsIt is possible that if you try experimenting in your REPL you might get a better understanding of the difference between the two methods.
Something like below could be a good start: