Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial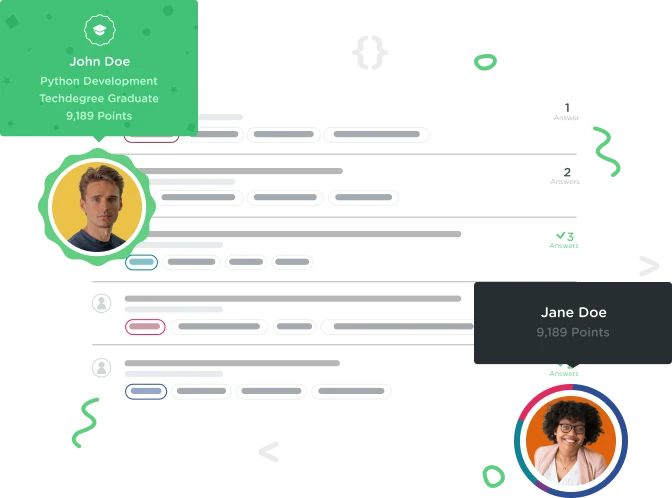

Blaine Fallis
2,449 Pointswhat's the colon doing? .Failure(let success):
here's the code in question:
enum Status {
case Success(String)
case Failure(String)
}
let downloadStatus = Status.Failure("Network connection unavailable")
switch downloadStatus {
case .Success(let success):
println(success)
case .Failure(let failure):
println(failure)
}
I get that downloadStatus is of type Status so you can shortcut that. What I'm unsure of is the syntax of (let failure): and also I need to review capitalization. Status is an enum type, downloadStatus a variable of type Status. what is failure with small f?
2 Answers

Chris Shaw
26,676 PointsHi Blaine,
This can be a bit tricky since we learned that let
checks for optionals and also defines a constant.
So what is it doing in the case of the enum
and the switch
?
Well it's assigning a value to a new variable which is something we've done many times in the past through previous Swift courses, just in this case it doesn't look right as we've never use it within a case statement before.
Let's break this down.
- You open your case statement and assign a value from the
Status
enum - next you open your parenthesis (optional)
- now you use a constant assignment to get the
String
value assigned to theenum
- you now have access to that
String
value within the case statement
Still confusing?
It will become clearer over time, essentially the constant assignment can be called anything you want so in the case of failure
we could call that wantWentWrong
and print that out using our println
statement.
Also as I said above enum
values are optional so we can for instance remove (String)
from our Success
case and then just check for Success
in our switch
case, in doing this we would need to change our println
statement to reference either a static string or a variable defined elsewhere but the overall code would work the same way.
enum Status {
case Success
case Failure(String)
}
let downloadStatus = Status.Success
switch downloadStatus {
case .Success:
println("The download was successful")
case .Failure(let failure):
println(failure)
}
Hope that helps, let me know if it's still confusing.
One more thing.
If we assign the type Status
to our downloadStatus
constant we can then remove the static reference to Status
in the value assignment.
let downloadStatus: Status = .Success

Blaine Fallis
2,449 Pointsactually, I do read here that the colon is just built into the grammar of a switch statement: switch control expression {
case pattern 1:
statements
case pattern 2 where condition:
statements
case pattern 3 where condition,
pattern 4 where condition:
statements
default:
statements
}
but with all the new types of expressions I'm learning you start to wonder, is the colon strictly part of the enums and associated values? But actually, it's just part of the switch statement grammar, as expected. We just happen to be using it here as part of an enum associated value. So it helps to review the basic syntax to narrow down why statements look the way they do. I was just trying to get down to the colon and why it's there, and discovered it is built into a switch statement. simple
But the let statement is also a cool topic, and optionals.