Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial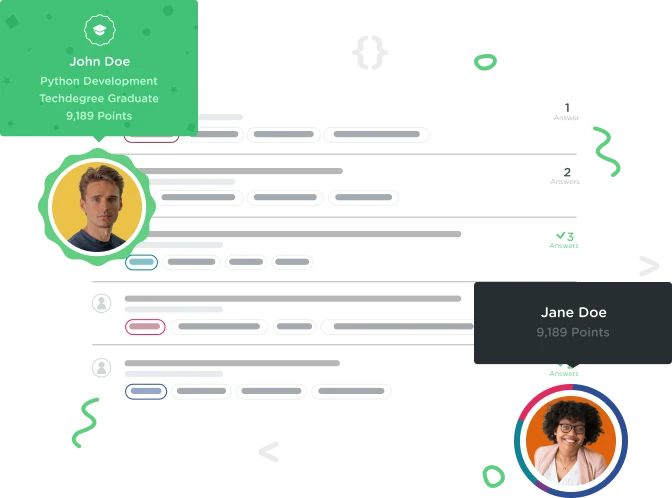
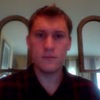
Casey Huckel
Courses Plus Student 4,257 PointsWhat's missing here?
Get 7 errors here and don't know why. I'll post compilation errors in addition to code.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
import java.util.Date;
import java.util.HashSet;
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> counts = new HashMap<>();
for (BlogPost mPost : mPosts) {
String category = mPost.getCategory();
Integer count = counts.get(category);
if (count == null) count = 0;
count++;
counts.put(category, count)
}
return counts;
}
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
1 Answer
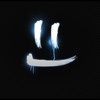
Grigorij Schleifer
10,365 PointsHi Casey,
delete the second imports of Map and HashMap.
Let us look at your code:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> counts = new HashMap<>();
for (BlogPost mPost : mPosts) {
String category = mPost.getCategory();
Integer count = counts.get(category);
if (count == null) { // added a curly bracket to open the if loop
count = 0;
}
count++;
counts.put(category, count); // added a semicolon here
} // added a curly bracker to close the for loop
return counts;
}
You was incrementing count only if it is null, not for every time you encounter the category. And you added category + count if the count was null. So I moved
count++;
counts.put(category, count);
out of the if statement.
I hope it help you a little bit.
Grigorij
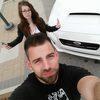
Jordan Ernst
5,121 Pointsdon't forget >>
Map<String, Integer> counts= new HashMap<String, Integer>();
Casey Huckel
Courses Plus Student 4,257 PointsCasey Huckel
Courses Plus Student 4,257 PointsHere's a list of errors:
./com/example/Blog.java:13: error: class, interface, or enum expected public Map getCategoryCounts() { ^ ./com/example/Blog.java:15: error: class, interface, or enum expected for (BlogPost mPost : mPosts) { ^ ./com/example/Blog.java:17: error: class, interface, or enum expected Integer count = counts.get(category); ^ ./com/example/Blog.java:18: error: class, interface, or enum expected if (count == null) count = 0; ^ ./com/example/Blog.java:19: error: class, interface, or enum expected count++; ^ ./com/example/Blog.java:20: error: class, interface, or enum expected counts.put(category, count) ^ ./com/example/Blog.java:23: error: class, interface, or enum expected } ^ Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 7 errors