Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial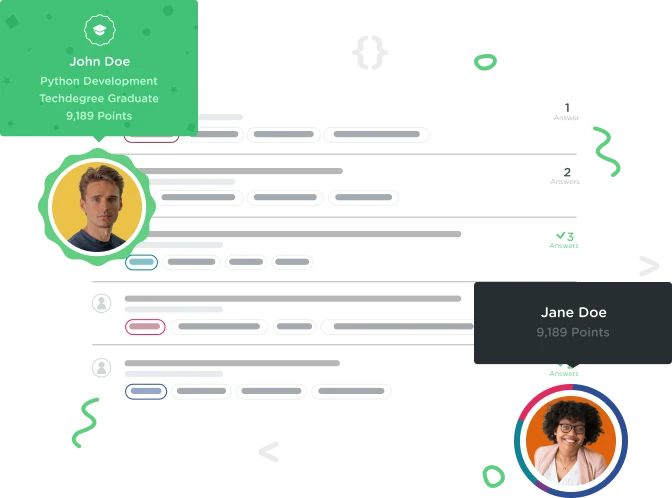
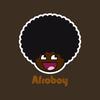
adsdev
15,583 PointsWhat's de deal with the && and the "and" operator ?
I kind of get what the && and "and" operators do. But also i kind of don't. I don't really get the logical thought behind it.
<?php
//output is false, because the following happens ($var1= (true && false));
$var1 = true && false;
//output is true, because the following happens ($var 2= true) and false);
$var2 = true and false;
php codesnippet won't work with me ... back to the question, can someone elaborate on this ? Thanks in advance :)
8 Answers

Cory Norell
10,613 PointsI just spent 10 minutes trying to understand this, and once I did I realized none of the answers here sufficiently hit the mark.
What is happening is this... Let's say we have the following code:
<?php
$var1 = true and false; // the value TRUE is assigned to $var1, the rest of the line is then ignored.
var_dump($var1); //var_dump simply dumps the value you had assigned to the variable above, which was TRUE.
$var2 = false and false // now the value FALSE is assigned to $var1, and the rest of the line is ignored.
// so when we var_dump...
var_dump($var2);
// it will now return false, because the rest of the code after the initial assignment of the first boolean to the variable is all ignored. Like it was never there.
?>
So just to really drive this home, we can use a couple extreme examples:
<?php
$foo = 1 and true and false and false or false and true or false and true;
// this looks outrageous, but var_dump will simply return the integer value 1.
// You can visualize what is happening behind the scenes like this:
$foo = 1 //and true and false and false or false and true or false and true;
// or an even more outrageous example...
$bar = false and true and potato and broccoli and this_is_insane and what_is_going_on and aowidjaowubdaowiuhd;
// you can run the above code just fine, because the system assigns the value FALSE to $bar, and then ignores the rest of the line.
?>
Hopefully that helps. I realize this is an old thread, but I'm sure many people overthink this as I did and miss what is going on. They are trying to finish the logical evaluation - as I was doing - and that makes no sense.
Someone please correct me if I'm wrong, but this works in every scenario I can think of.

filipepacheco
Full Stack JavaScript Techdegree Student 21,416 PointsHave a look into operator precedence. Here is link to the manual.
Here is a copy of an user contribution note there:
Watch out for the difference of priority between 'and vs &&' or '|| vs or':
<?php
$bool = true && false;
var_dump($bool); // false, that's expected
$bool = true and false;
var_dump($bool); // true, ouch!
?>
Because 'and/or' have lower priority than '=' but '||/&&' have higher.

Sulaiman Noh
14,538 PointsWhy do I think this is ridiculous? If the 'and' is lower than '=' precedence. What happened to the false statement after the ($bool = true)? is it ignored?
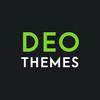
Alexander Samokhin
9,647 PointsI also little bit confused about it. Can someone explain?
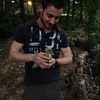
John Ireland
6,585 PointsMy question is, how common are values like this in a 'real job scenario'? I'm only reviewing php here, and so far(in my very limited experience) I've never come across an issue like this yet. Is this one of those 'just so we know' things?

Collin Berg
33,471 PointsIf you ever come across this debugging and you can't find why a value is being reassigned this might be cause. I typically only use || and && in my day to day. I think they just want students to be aware of the difference, but suggest using the symbols, over the word forms.
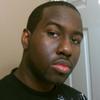
stefon matthew
4,240 PointsThe = sign that comes before the and/or has a higher precedence/importance so the and/or can't change the value of the statement.

john culjak
Courses Plus Student 4,074 PointsCory's answered filled the hole I was having. It didn't make sense how the variables were being evaluated. After reading his post it became more clear. But after reading Kat's I'm still a bit unsure about what qualifies something to be true or false.
For example why are both of these true?:
var_dump ((bool) 1); // bool(true) var_dump ((boo) -2); // bool(true)

FREDY AREVALO
785 PointsIt is all on operator precedence. && operator has a more precedence than AND operator. When && is used is the same as using parenthesis to establish order of operation. example:
3*(1+2)= since parenthesis are used addition will be perform first. it is the same as when we used && operator. I hope it helps. Take time to read php manual
regards
-f
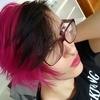
Kat Cuccia
786 Points$a = true && $b = true
will evaluate to true.
$a = true && $b = false
will evaluate to false
$a = false && $b = true
will evaluate to false
$a = false && $b = false
will evaluate to false.
When using an AND (&&) operator, it requires that ALL CONDITIONS evaluate to true before outputting an overall true or false. OR (||) only requires that part of the input evaluate to true in order to output true.
Alexander Samokhin
9,647 PointsAlexander Samokhin
9,647 PointsYes, but you forgot about difference between AND and && operator. That was the point of this topic.
Cory Norell
10,613 PointsCory Norell
10,613 PointsYes, you're right - I apologize. I suppose I should have specified, but I thought that was very clear in the video and the other responses to this question. The thing that was not clear is why the return value of var_dump was what it was.
The thing I was attempting to answer - the thing that I would bet confuses the vast majority of people who are confused about this topic -is that everything after the initial assignment operation is ignored. I looked in various places (Wikipedia, W3Schools, and Stackoverflow), and in all those places it was abundantly clear that AND has a lower precedence than = and &&. But it was not mentioned in any of those places exactly why we get the result we do.
Perhaps this is all superfluous, but I think not.
Marco Fregoso
500 PointsMarco Fregoso
500 PointsI really got it now :D thank you so much! I burst out laughing at your examples lol. Surprisingly the && and || operators work the same as in java and javaScript, so there wasn't any problem understanding that
drawgo
3,205 Pointsdrawgo
3,205 PointsThe rest of the line is not ignored (unless there is some optimization I'm not aware of, in fringe situations).
An extreme example:
General expression order clear-up:
Corrections are welcome.
Juan Rivera
PHP Development Techdegree Student 960 PointsJuan Rivera
PHP Development Techdegree Student 960 PointsThis really helped me understand. Thanks!!