Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial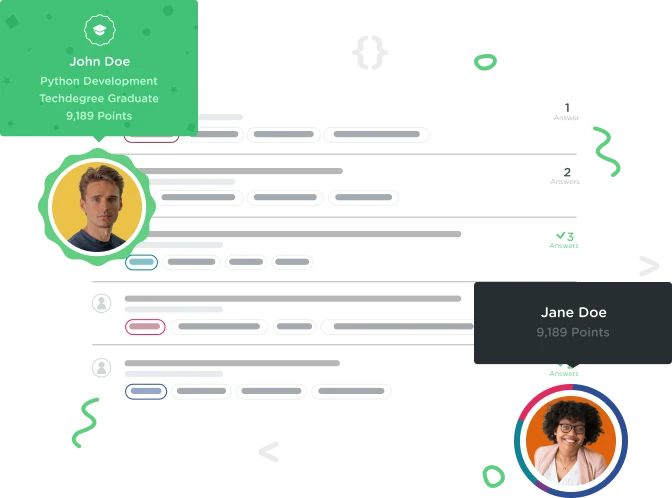

Matt Bloomer
10,608 Pointswhat to do
I am not exactly sure how to go about this; can anyone help?
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
2 Answers
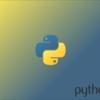
Kevin Faust
15,353 PointsHey Matt,
I redid that challenge to rejog my memory and I remember it took me a long time the first time. I'll break it down in a easy way. So let's start with the first step:
So while we have no customer support reps in our queue, we want music to play. We can use a while loop for this easily. Use a while loop to check if our queue has nothing in it. Check the java docs on queues. It only has 6 methods and you'll be able to see what you gotta use. And then inside that while loop, just call the music method! Ill let you try this first and you can look at my answer at the bottom if your still confused.
Ok onto the second step. So for this step, we have finally got our customer rep as it passed that while loop. So lets take the first person out of the queue. Do you remember which method you gotta use? Once we pull the person out of the queue, let's assign to this variable that is just chillin at the top of the method. It looks like this:
CustomerSupportRep csr;
so take the person from the queue and assign it to that. Now we can reference our customer support easily by just typing "csr". So once we got our "csr", lets call the method that does the assisting and pass in the customer.
Now last step! yay lol
So all we do here is pop that "csr" back into the queue. I think you can do that easily
I think you can do it now but i have posted my solution in the bottom if your still stumped. let me know if your still confused.
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while (mSupportReps.peek() == null) { // or we can use .poll() as they both return null if theres nothing in there
playHoldMusic();
}
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr = mSupportReps.poll();
csr.assist(customer);
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
Hope this cleared everything up. Cheers!

Matt Bloomer
10,608 PointsThanks for all the help. I pretty much got the first part, but was getting bogged down on the second part.
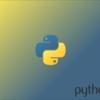
Kevin Faust
15,353 Pointsthank me with points ;)