Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial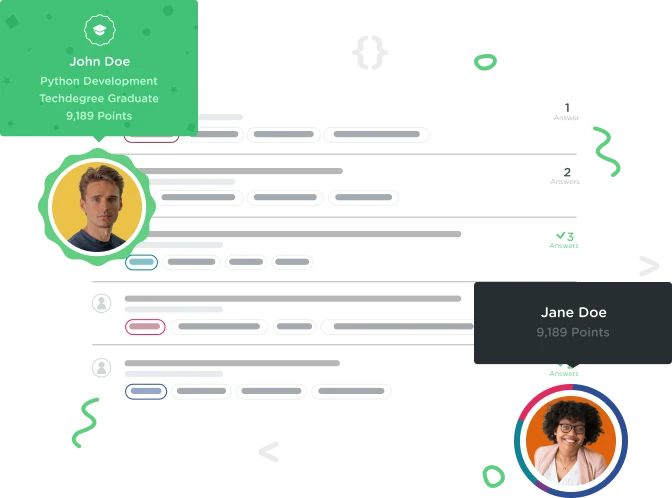

Mike John
3,182 PointsWhat mistake am I doing in this code.?
What mistake am I doing in this code.?
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<ul>
<li id="first">First Item</li>
<li id="second">Second Item</li>
<li id="third">Third Item</li>
</ul>
<script src="app.js"></script>
</body>
</html>
let myList = document.querySelector("ul");
let firstListItem= document.querySelector("#first");
myList.removeChild('#first');
2 Answers
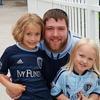
Nick Trabue
Courses Plus Student 12,666 PointsYou're selecting the first item with
let firstListItem = document.querySelector('#first');
and then telling it to remove "#first" instead of using the variable you declared
let myList = document.querySelector('ul');
let firstListItem = document.querySelector('#first');
myList.removeChild(firstListItem);
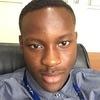
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsHi Mike, most of your code is good. It's just the last line. You have to declare 'myList' variable as the parent of the 'firstListItem' variable before you can call the removeChild() method. And you don't call it with '#first' as an argument, that's a CSS selector you used in the previous line to select the first item on the list and stored it in the 'firstListItem' variable.
let firstListItem= document.querySelector("#first");
myList.removeChild('#first');
So you just call the removeChild() method passing in the 'firstListItem' variable and not that CSS selector.
let myList = document.querySelector("ul");
let firstListItem= document.querySelector("#first");
myList = firstListItem.parentNode;
myList.removeChild(firstListItem);
A bonus tip is to use the keyword 'const' when selecting and storing elements in a DOM page rather than 'let'.
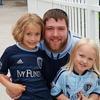
Nick Trabue
Courses Plus Student 12,666 PointsYour last suggestion is great, this would be a perfect time to use const.
You don't have to set myList as the parent of myfirstListItem. Javascript determines parent nodes based on html structure.
<ul id="some-id">
<li id="first">Some item</li> <!-- Parent is ul with id of some-id -->
<li>Some other item</li> <!-- Parent is ul with id of some-id -->
</ul>
<ul id="other-id">
<span class = "coolspan">
<li>Another list</li> <!-- parent is span -->
</span>
</ul>
I think the ideal way to do this, if you were going to have multiple lists on a page, is to give your target list an ID and targeting myList via id
const myList = document.getElementById('some-id')
const firstListItem = document.getElementById('first')
myList.removeChild(firstListItem);
In fact, if he changed the keyword to const like you suggested, he would get an error when setting myList = firstListItem.parentNode. When you do that, you're trying to change the value of myList and const variables cannot be overwritten in the same block.
const myList = document.querySelector('ul')
//other stuff
myList = firstListItem.parentNode // <--this will create a console error and anything below it will not run