Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial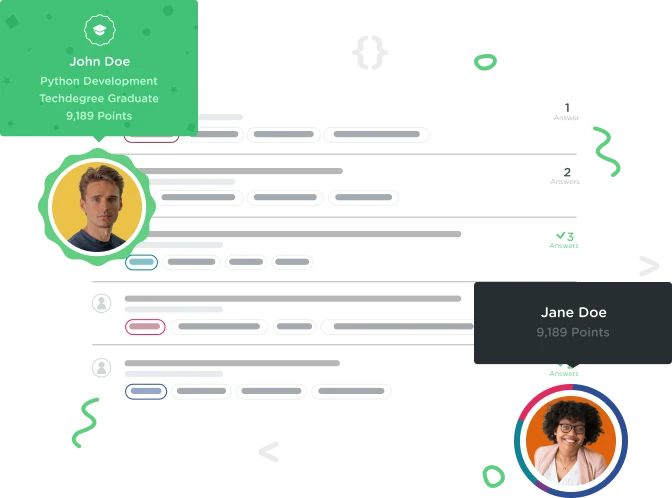

Mamta Chaudhary
527 PointsWhat is wrong with my program?
I am new to java and I am writing this program to output the guest's information which is private. So I created two java classes and made the variables private in the Guest.java Class and I used the setter and getter methods to use the private variables, but in the other Main.java Class it seems to not be working.
package Motel; import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Guest Identification = new Guest();
Identification.Guest();
System.out.println("Welcome to the Motel");
System.out.println("Please answer the following Questions to Check In: ");
System.out.println("Enter your Full Name: ");
String name = input.nextLine();
System.out.println("Enter your Address: ");
String address = input.nextLine();
System.out.println("Date of Birth: ");
int dateOfBirth = input.nextInt();
System.out.println("Enter your Identification Card Number or SSC number: ");
int iD = input.nextInt();
System.out.println("Enter your Name for a E-Signature: ");
String signature = input.nextLine();
System.out.println("Please Make sure this information is Correct: ");
System.out.printf(" Name: %s %n",Identification.getGuestName());
System.out.printf(" Address: %s %n",Identification.getGuestAddress());
System.out.printf("D.O.B: %d %n",Identification.getGuestDateOfBirth());
System.out.printf("ID#: %d %n", Identification.getGuestIdentificationNumber());
System.out.printf("E Signature: ",Identification.getGuestSignature());
}
}
package Motel;
public class Guest {
private String guestName;
private String guestAddress;
private String guestSignature;
private int guestDateOfBirth;
private int guestIdentificationNumber;
public Guest (String guestName, String guestAddress, String guestSignature,
int guestDateOfBirth, int guestIdentificationNumber) {
this.guestName = guestName;
this.guestAddress = guestAddress;
this.guestSignature = guestSignature;
this.guestDateOfBirth = guestDateOfBirth;
this.guestIdentificationNumber = guestIdentificationNumber;
}
public String getGuestName () {
return guestName;
}
public void setGuestName (String name){
guestName = name;
}
public String getGuestAddress () {
return guestAddress;
}
public void setGuestAddress (String address){
guestAddress = address;
}
public String getGuestSignature () {
return guestSignature;
}
public void setGuestSignature (String signature){
guestSignature = signature;
}
public int getGuestDateOfBirth () {
return guestDateOfBirth;
}
public void setGuestDateOfBirth ( int dateOfBirth){
guestDateOfBirth = dateOfBirth;
}
public int getGuestIdentificationNumber () {
return guestIdentificationNumber;
}
public void setGuestIdentificationNumber ( int iD){
guestIdentificationNumber = iD;
}
}
1 Answer

Emmanuel C
10,636 PointsThe Guest class only has one constructor that takes in a bunch of arguments, when you create the Guest object in the main method, you don't provide the parameters that the only constructor it has, needs. So you are basically calling an undefined method, since a constructor with no arguments doesn't exist.
Also when you use Identification.Guest(); There is no Guest() method in your Guest class, so that's coming up as another undefined error. You can create a blank constructor that takes no arguments, and have the other constructor as an overload
public Guest() {}
After adding that blank constructor and commenting out that Identification.Guest(), since I'm not sure what you want to do with that Guest() method, your code works.
Two things I've noticed though is, be careful with the date of birth, you're using nextInt(), and if they put in 01/01/1990, then an error will be thrown as the forward slash "/" are not considered ints. I suggest getting input as a string and then converting it to a Date object, from java.util.Date.
Second is that you don't assign those inputs into your object so when the user enters their info and you output their info to make sure its correct, it will all come out blank, since you're saving the inputs in local variables but outputting the values from the Guest object using the getter. I suggest using the Guest object setter when the user inputs those values. That way it will be stored within the Guest object.