Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial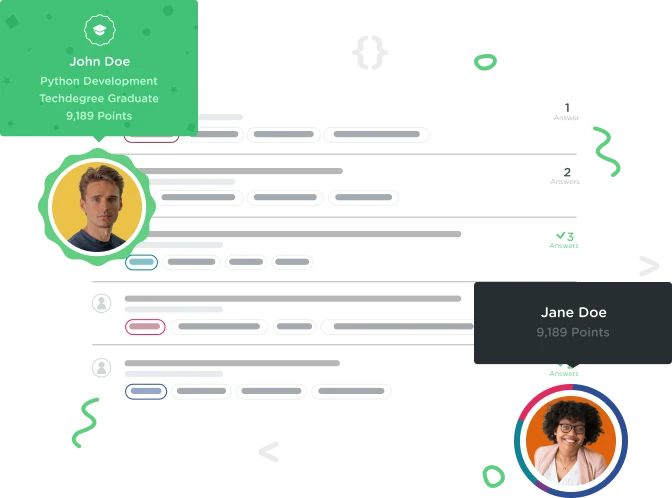

amnah ilyas
5,726 PointsWhat is wrong with my code?
now let's add a method named run_lap. It'll take a length argument. It should reduce the fuel_remaining attribute by length multiplied by 0.125. Oh, and add a laps attribute to the class, set to 0, and increment it each time the run_lap method is called.
class RaceCar:
#laps=0
def __init__(self,color,fuel_remaining,**kwargs):
self.color=color
self.fuel_remaining=fuel_remaining
self.laps=0
for key,value in kwargs.items():
setattr(self,key,value)
def run_lap(self, length):
self.fuel_remaining -= length * 0.125
self.laps+=1
11 Answers
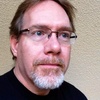
Chris Freeman
Treehouse Moderator 68,441 PointsYou are on the right path. In addition to assigning a value to self.laps
, the code needs to allow the laps to be set to a specific value.
- Add a
laps
keyword to the__init__
method. Include a default value. The value zero you used before works fine. - set
self.laps
to thelaps
argument
Post back if you need more help. Good luck!

Pratham Patel
4,976 PointsI tried what you said with her code but i still got errors
class RaceCar:
def __init__(self,color,fuel_remaining,**kwargs, laps=0):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key,value in kwargs.items():
setattr(self,key,value)
def run_lap(self, length):
self.fuel_remaining -= length * 0.125
self.laps += 1
[MOD: added ```python formatting -cf]
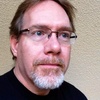
Chris Freeman
Treehouse Moderator 68,441 PointsPratham Patel, you are very close! The laps
keyword assignment must come before the generic kwargs
catch-all parameter.

amnah ilyas
5,726 Pointsclass RaceCar:
def __init__(self,color,fuel_remaining,**kwargs):
self.color=color
self.fuel_remaining=fuel_remaining
self.laps=0
for key,value in kwargs.items():
setattr(self,key,value)
def run_lap(self, length):
self.fuel_remaining -= length * 0.125
self.laps=self.laps+1
This code works!!

Harihar Kandula
6,486 Pointswhy should the length not be self.length ?
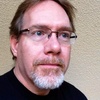
Chris Freeman
Treehouse Moderator 68,441 PointsThe perimeter length
is a local variable to the method run_lap
. It is not an attribute to the class RaceCar
.
As a local variable, it may be accessed directly and is not referenced as an attribute.
Post back if you need more help. Good luck!!!

Chad Goldsworthy
4,209 PointsJust for anyone still struggling with this, I think I managed to find the issue. I tried all the code other people posted and said worked, but it did not work for me (and it seems there were many people having the same issue).
I ran it through my IDE and realised the length*0.125 in the run_lap method was causing a TypeError: unsupported operand type(s) for -=: 'str' and 'float'
So, when assigning fuel_remaining in the init method, you should convert it to a float as well, like: self.fuel_remaining = float(fuel_remaining)
I say "you should" lightly though, I'm no expert but it's just what fixed the issue for me.
This is the code that eventually worked for me:
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = float(fuel_remaining) # <-- this is what fixed it for me,
# adding the float() method
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= (length * 0.125)
self.laps += 1
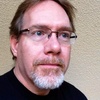
Chris Freeman
Treehouse Moderator 68,441 PointsUsing float()
shouldn’t be necessary. I suspect the TypeError was caused by the arguments chosen during your IDE testing.
As is, this code doesn’t pass the challenge. It’s missing the lap
parameter in the __init__
method.
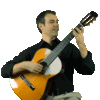
Stephen Hanlon
11,845 PointsNot having any luck with this code. What am I doing wrong?
class RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= self.length * 0.125
self.laps += 1
I also tried this but no luck either:
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= self.length * 0.125
laps += 1
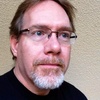
Chris Freeman
Treehouse Moderator 68,441 PointsIn the first code sample
# should be
self.fuel_remaining -= length * 0.125
# instead of
self.fuel_remaining -= self.length * 0.125
Same for the second code sample, plus
# should be added to __init__
self.laps = 0
# in run_lap should be
self.laps += 1
# instead of
laps += 1
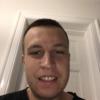
Andrii Gorokh
14,809 PointsWhy this doesn't pass?
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
fuel_remaining -= length*0.125
self.laps = self.laps+1
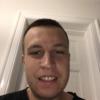
Andrii Gorokh
14,809 Pointsok got it, I missed self.fuel_remaining....
Could someone explain in detail what exactly self does?
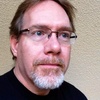
Chris Freeman
Treehouse Moderator 68,441 PointsIn a class method, the parameter self
gets assigned to the instance that is being referenced so that the code knows where to find the attribute to update.

Alex Brooklyn
7,238 Pointsdef __init__(self, color,fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for key, value in kwargs.items():
setattr(self,key,value)
def run_laps(self, length):
self.fuel_remaining -= self.length * 0.125
self.laps = self.laps + 1
Don't really get what is wrong here! Please advise
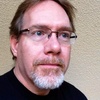
Chris Freeman
Treehouse Moderator 68,441 PointsYou are very close.
- The method name should be
run_lap
notrun_lap
s - refer to the parameter
length
and not the non-existent attributeself.length
Post back if you need more help! Good Luck!!

Jamar Slade
2,480 PointsChris Freeman how come here we can the length attribute as just "length" opposed to "self.length" like we have with every other attribute?

Ashraf Nabil
6,633 PointsSharing the correct code:
class RaceCar:
def __init__(self,color,fuel_remaining,laps=0,**kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self,length):
self.laps += 1
self.fuel_remaining -= length * 0.125
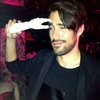
Adrian Torrente Tenreiro
12,540 Pointsclass RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
#reduce the result of the **
laps += 1
self.fuel_remaining -= length * 0.125
Do you guys see any issues???
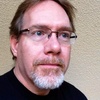
Chris Freeman
Treehouse Moderator 68,441 PointsYou're VERY close! change laps += 1
to self.laps += 1
Post back if you need more help. Good luck!
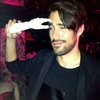
Adrian Torrente Tenreiro
12,540 PointsFixed
Ivan Frances Alcantara
4,528 PointsIvan Frances Alcantara
4,528 PointsI copy and paste Amnah's code and it accepts it as correct. Does self.laps=0 already create a keyvalue argument through **kwargs?