Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial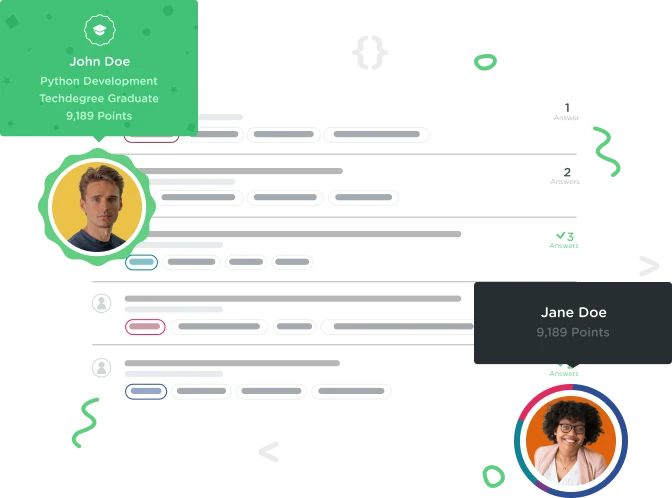

Cyrille Turnier
1,715 PointsWhat is wrong with my code?
For each case in the switch statement, I read from the dictionary like the example we used in class for the airportCodes.
Can you please let me know why the code isnt compiling?
Thanks,
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels", //
"LIE": "Vaduz", //
"BGR": "Sofia", //
"USA": "Washington D.C.", //
"MEX": "Mexico City", //
"BRA": "Brasilia", //
"IND": "New Delhi", //
"VNM": "Hanoi"]
for (choice) in world {
// Enter your code below
switch (choice) {
case world["BEL"]: europeanCapitals.append("Brussels")
case world["LIE"]: europeanCapitals.append("Vaduz")
case world["BGR"]: europeanCapitals.append("Sofia")
case world["IND"]: asianCapitals.append("New Delhi")
case world["VNM"]: asianCapitals.append("Hanoi")
case world["USA"]: otherCapitals.append("Washington D.C.")
case world["BRA"]: otherCapitals.append("Brasilia")
case world["MEX"]: otherCapitals.append("Mexico City")
}
}
1 Answer

robertrinca
Courses Plus Student 11,316 PointsWell, first of all your switch statement isn't exhaustive (need a default case in there).
Second, on the loop, you want to go through the key and the value. So each run through the switch statement, airportCode contains the key of the array, and the city contains the value of the array.
Third, in the case statements, just look for the strings you need, you don't need to put the array name in there.
So, to make your code go, you could use below:
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels", //
"LIE": "Vaduz", //
"BGR": "Sofia", //
"USA": "Washington D.C.", //
"MEX": "Mexico City", //
"BRA": "Brasilia", //
"IND": "New Delhi", //
"VNM": "Hanoi"]
for (airportCode, city) in world {
// Enter your code below
switch (airportCode) {
case "BEL": europeanCapitals.append(city)
case "LIE": europeanCapitals.append(city)
case "BGR": europeanCapitals.append(city)
case "IND": asianCapitals.append(city)
case "VNM": asianCapitals.append(city)
default: otherCapitals.append(city)
}
}
Or if you want to make it even a little more compact, put multiple arguments on the case, so it would look like this:
for (airportCode, city) in world {
// Enter your code below
switch (airportCode) {
case "BEL", "LIE", "BGR": europeanCapitals.append(city)
case "IND", "VNM": asianCapitals.append(city)
default: otherCapitals.append(city)
}
Hope that helps.