Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial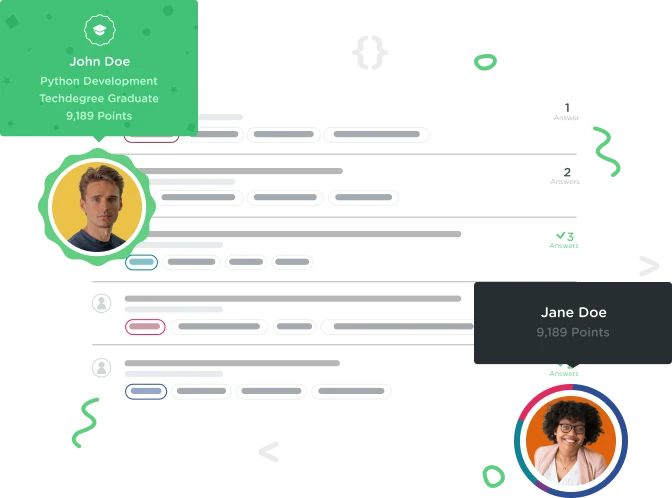

Jay Radia
2,919 Pointswhat is wrong with my code?
it keeps saying "Make sure the value you are assigning to greeting is an interpolated string" my output is correct and reads "Hi there, Jay. How are you?" but it's not letting me pass the task. Have I incorrectly set out my code?
// Enter your code below
let name = "Jay."
let hello = "Hi there, "
let string = " How are you?"
let greeting = "\(hello) \(name)" + "\(string)"
4 Answers
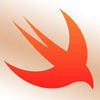
Jeff McDivitt
23,970 PointsYou need to concatenate the last part
let name: String = "Jeff"
let greeting = "Hi there, \(name)"
let finalGreeting = "\(greeting)" + "How are you?"

andren
28,558 PointsTreehouse challenges are pretty picky about how you write and format strings. They often require that your string match the provided example to the dot. The greeting the task asks for only includes "Hi there, NAME" and nothing beyond that. By adding more to the greeting your code will be marked as wrong.
Also you don't need to split the greeting into so many variables. An interpolated string can contain both regular text and placeholders. So you can achieve the task with only two lines of code like this:
let name = "Jay"
let greeting = "Hi there, \(name)"
It's also worth noting that using the + operator is an example of string concatenation rather than string interpolation. So you use both string interpolation and string concatenation in your code. Which is not really a problem normally (though a bit redundant) but for this challenge they specifically want you to use string interpolation only.
Edit: Added note about string concatenation.

Jay Radia
2,919 Pointslet name = "Jay"
let finalGreeting = "How are you?"
let greeting = "Hi there, \(name). \(finalGreeting)"
So i've changed my code to this but it needs to be concatenated. The error shows as: make sure you're using concatenation to create the final string value required!.
how do I change it to the correct format?

Noah Muhammad
Courses Plus Student 641 Pointslet name = "Birdy" let greeting = "Hi there , " let interpolatedGreetings = "(greeting),(name)" Please assist with what I'm doing incorrectly

Jay Radia
2,919 PointsWrite it out as follows and it should work, let me know how it goes.
let name: String = "Birdy"
let greeting = "Hi there, \(name)"
let finalGreeting = "\(greeting)" + "How are you?"

Jay Radia
2,919 Pointsit said on the task to use the constant finalGreeting so had to name it that.
And you don't need to write out string with the semi colon because Swift automatically recognises the type you are using, I just did it for practise.
Jay Radia
2,919 PointsJay Radia
2,919 PointsThat's great thank you it worked!
Noah Muhammad
Courses Plus Student 641 PointsNoah Muhammad
Courses Plus Student 641 PointsThanks your code helped a lot but I don't understand why we used final instead of interpolated and why you said name: and then type string?