Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial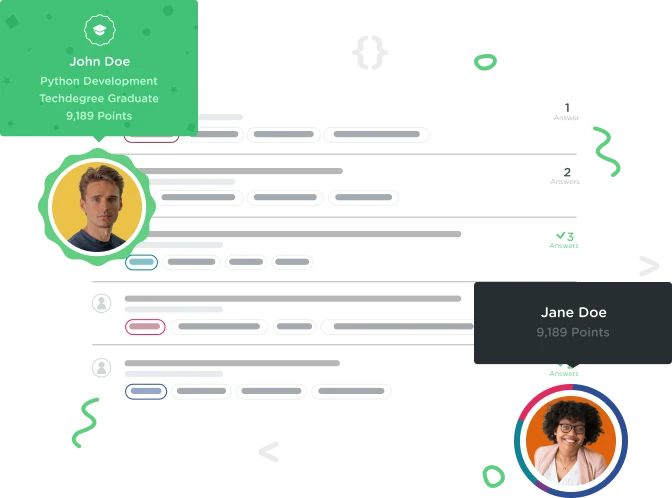

Unsubscribed User
Courses Plus Student 2,233 Pointswhat is the point
I do not know what this program wants
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double, description: String){
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = description
}
}
7 Answers
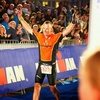
Steve Hunter
57,712 PointsOK - that's a good start.
First, though, you don't need to pass the description
in as a parameter. I've mentioned this in my previous posts. The user controls the color parameters, the description
is made up from those. It is not user defined.
We start with:
init(red: Double, green: Double, blue: Double, alpha: Double){ // <-- no description here
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
}
}
So, you have passed in four Double
values. Those values are used in making the description
string.
The string needs to say "red: *red parameter value*, green: *green value parameter, blue: *blue parameter value, alpha: *alpha value parameter*
"
How do you incorporate, or interpolate, values into a string?
If you set the description
string to be equal to the above string with the right interpolated values (the ones you've passed into the init
method) then you're done.
init(red: Double, green: Double, blue: Double, alpha: Double){ // <-- no description here
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: *red parameter value*, green: *green parameter value*, blue: *blue parameter value*, alpha: *alpha value parameter*" // <-- use the \(var_name) format, yes?
}
}
Let me know how you get on!
Steve.
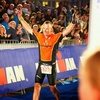
Steve Hunter
57,712 PointsHi there,
You're nearly there with that - very close!
A couple of things. Don't assign the number values to the attributes inside the init
method. Those numbers are just examples. You have passed values into the init
method for each of them. Assign those values inside the method. So, you've passed in red: Double
into the method. Inside init
use self.red = red
to use that passed-in value.
Lastly, the description
attribute doesn't need passing into init
but it does need to be set inside init
. Have a look at the challenge and use string interpolation to construct a string that's the same as requested in the challenge. Something like "red: \(red), green: \(green) ...
" I'll leave you to do the rest!
Let me know how you get on.
Steve
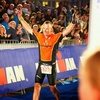
Steve Hunter
57,712 PointsHi again,
What's the issue with description
? Remember, you don't need to pass it into the init
method. But, as with all stored properties, it does need to be set to a value within init
.
The description
is a string made up, in part, of the other stored properties that you do pass into the init
method. Those properties define the color being created; description
does not, it is just that, a string that describes the color values.
Don't worry about 'memberwise'; that's just a technical term that describes how the initializer works through the stored properties. Have a quick look at the docs for more on that.
If you're still stuck, post your code and I'll take a look.
Steve.

Unsubscribed User
Courses Plus Student 2,233 PointsThanks, I almost understand the work of init , but the problem in String(description) and something else I did not understand what (membereise) means .

Unsubscribed User
Courses Plus Student 2,233 PointsI'm sorry, I have tired you with me, but I could not understand how to solve the String(description)
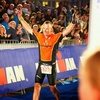
Steve Hunter
57,712 PointsOK. I'll walk you through it - post your current code and we can go from there!

Unsubscribed User
Courses Plus Student 2,233 Pointsstruct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double, description: String){
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
}
}

Unsubscribed User
Courses Plus Student 2,233 Pointsthanks , it's done , I have tired you with me .
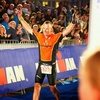
Steve Hunter
57,712 PointsGood work! Glad you got it sorted! I'm happy to help.