Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial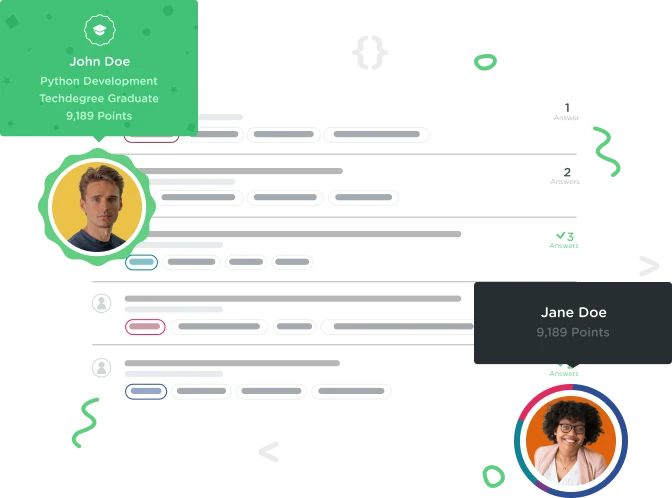

liamthornback2
9,618 PointsWhat is the hashCode() method for?
I've taken a few Treehouse courses on Java before and this is the first time I've seen that method added to a Java class.
2 Answers
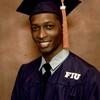
Dane Parchment
Treehouse Moderator 11,077 PointsTo add-on to Steven Parker's answer, hashCode()
is extremely useful if you want a custom class/object to be utilized in a HashTable/HashMap of any sort. This is because a hashing function is used to determine the position of an object within the hashtable, and effective hash functions will stave off collisions and keep the hashtable/hashmap perform-ant.
For example let's assume we have the following class that represents a User in an application:
public class User {
private long id;
private String firstName;
private String lastName;
private int age;
// standard getters/setters/constructors
}
If we want to use this user within a hashtable it needs to have a hashcode. (Normally this is done by default via a general hashing function, but you may want to customize the function yourself to make it perform better).
In order to use the hashCode()
method we also need to implement the equals()
method as well, for reasons that Parker explained in his answer.
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null) return false;
if (this.getClass() != o.getClass()) return false;
User user = (User) o;
return id == user.id
&& (firstName.equals(user.firstName))
&& (lastNameName.equals(user.lastName))
&& (age.equals(age));
}
This does what it says on the tin, basically we check and see if our User object is equal to another User object.
With this done we can now implement the hashCode()
method.
@Override
public int hashCode() {
int hash = 7;
hash = 31 * hash + (int) id;
hash = 31 * hash + (firstName == null ? 0 : firstName.hashCode());
hash = 31 * hash + (lastName == null ? 0 : lastName.hashCode());
hash = 31 * hash + (int) age;
return hash;
}
Basically what this does is try to generate a unique hashCode for the User object. If you want me to explain why I used the hash function that I did, I will be glad to explain. But as a note for creating hash functions, use primes.
Anyway, this will allow your Object to be optimized for use in structures like Hashtables.
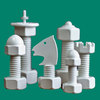
Steven Parker
241,970 PointsThe "hashCode" method is handy for determinng if an object has changed during the run of the program. It's also related to equality tests between objects. The official requirement is:
If two objects are equal according to the equals(Object) method, then calling the hashCode() method on each of the two objects must produce the same value