Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial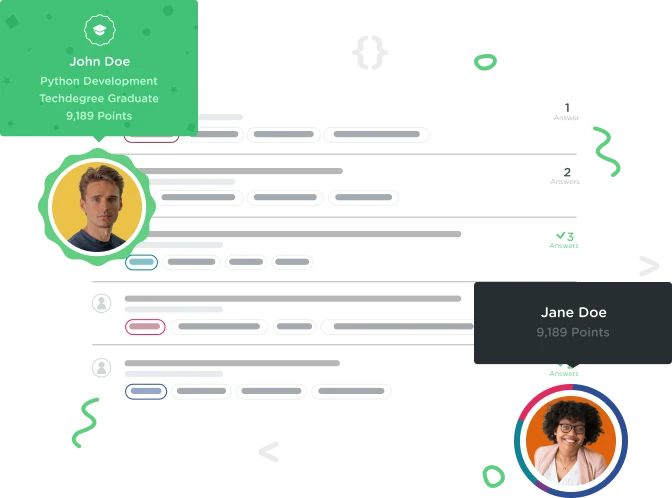

Curtis Preston
1,877 Pointswhat is a bad initializer in the for-loop. i'm writing it the exact same way we did in the example
i'm following the syntax as it was in the lesson, except i didn't define the variable type char again since it was already defined. the error is telling me that i have a bad initializer and i dont know what that is. as far as i can tell... the "initializer" is simply "for". thanks for your help.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) {
int count = 0;
for (letter : tiles.toCharArray()) {
++count;
}
return count;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer
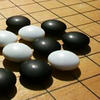
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsHello,
You're using a "for-each" loop. To understand why your code doesn't work, you need to understand how for-each loops work. A way to describe what your for-each loop does is this: "For each character in the 'tiles' String, do [whatever is in the loop]."
So it will always go through each character in the 'tiles' string. Each time the loop runs, the current character is stored in the char variable you define in the braces.
The variable before the colon is always a new variable that exists only within the for-each loop. You can't just use an existing variable, it won't work. The for-each loop will always go through each character in the 'tiles' string, and each time it will put the current character in the variable defined before the colon. So this variable belongs to the for-each loop only, it can't exist outside of the loop at all (it's not in scope).
What you're trying to do is to loop only through the characters that match the 'letter' variable. You can't do that with a for-each loop! It will always loop through all the characters. What you can do is to not increase the 'count' variable every time there is a loop, but only when the current character matches 'letter'. So you just add an if block in your loop.
public int getCountOfLetter(char letter) {
int count = 0;
for (char c : tiles.toCharArray()) {
if (c == letter) {
++count;
}
}
return count;
}
Let me know if you have more questions.
Curtis Preston
1,877 PointsCurtis Preston
1,877 Pointsthank you so much!