Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial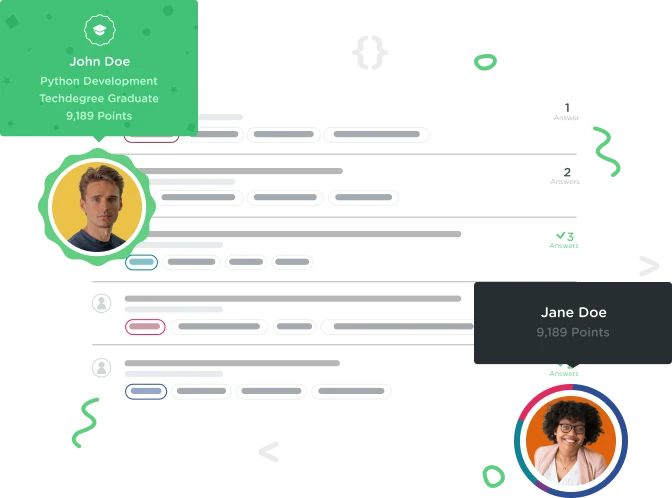

zang gransis
Courses Plus Student 436 Pointswhat i doing wrong??
why compiler not letting me add my name (as a rep) to the queue?
import java.util.ArrayDeque;
import java.util.Queue;
//This is a Call Center application.
//There is a group of Customer Support Representatives
//that assist Customers.
//When representatives become available they enter the queue.
//The acceptCustomer method gets called when a new customer phones in.
// I've left 3 things for you todo in the comments below.
//All other code is only for your reference, no need to change it.
public class CallCenter {
private Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
while(mSupportReps.size() < 0)
{
playHoldMusic();
}
if (mSupportReps.size() > -1)
{
csr = new CustomerSupportRep("zang");
csr.assist(customer);
mSupportReps.add("zang");
}
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
Henrik Hansen
23,176 Points csr = new CustomerSupportRep("zang");
csr.assist(customer);
// You are trying to add a string to the queue. I think you would want to add csr instead.
mSupportReps.add("zang");

zang gransis
Courses Plus Student 436 Pointsthanx. that worked! but now after fixing im getting this message:
"Bummer! Did you remember to call the assist method on the CustomerSupportRep?"
as you can see, i called the asssist method. so what do they want?
Henrik Hansen
23,176 PointsI dont really know what is wrong. But i don't really get what you are checking for in the if blocks here:
// If your queue is smaller than 0 ( -1 and below that is ). Try .size() < 1
while(mSupportReps.size() < 0)
{
playHoldMusic();
}
// here you are checking if queue is 0 or more. Try .size() > 0
if (mSupportReps.size() > -1)
{
csr = new CustomerSupportRep("zang");
csr.assist(customer);
mSupportReps.add("zang");
}

zang gransis
Courses Plus Student 436 Pointsi removed the whole if statement. still no luck!!
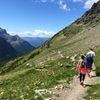
Stephen Wall
Courses Plus Student 27,294 Points// First we want to check the que and see if there are any support reps
// If not, play them some silky smooth hold music:
while (mSupportReps.peek() == null) {
playHoldMusic();
}
// Once we get a support rep, remove them from the queue and have them assist the customer:
csr = mSupportReps.poll();
csr.assist(customer);
// Finally after the customer has been assisted, add support rep back to the queue:
mSupportReps.add(csr);
Mirosław Sosnowski
1,402 PointsMirosław Sosnowski
1,402 PointsI guess you had in mind If-else statement if so check this out -->
public void acceptCustomer(Customer customer) { CustomerSupportRep csr; if (mSupportReps.peek() == null) playHoldMusic(); } else { csr = mSupportReps.poll(); csr.assist(customer); mSupportReps.add(csr); }