Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial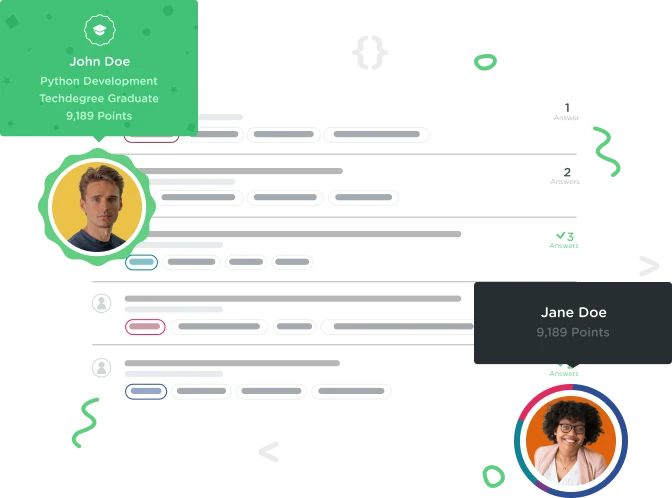
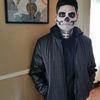
Jorge Otero
16,380 PointsWhat I did wrong?
This is my code:
private String normalizeDiscountCode(String discountCode) {
String codeUpperCase = "";
for (char codeDigit : discountCode.toCharArray()) {
if (!Character.isLetter(codeDigit) || codeDigit != '$') {
throw new IllegalArgumentException(codeDigit + " is not an authorize digit");
}
codeUpperCase += Character.toUpperCase(codeDigit);
}
return codeUpperCase;
}
This is the error I got:
Exception in thread "main" java.lang.IllegalArgumentException: v is not an authorize digit
at WorkspaceClass.normalizeDiscountCode(WorkspaceClass.java:6)
at Workspace.main(Workspace.java:6)
3 Answers
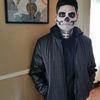
Jorge Otero
16,380 PointsUse && instead of || solve my problem. Note: This challenge is for the course Validating and Normalizing User Input
private String normalizeDiscountCode(String discountCode) {
String codeUpperCase = "";
for (char codeDigit : discountCode.toCharArray()) {
if (!Character.isLetter(codeDigit) && codeDigit != '$') {
throw new IllegalArgumentException(codeDigit + " is not an authorize digit");
}
codeUpperCase += Character.toUpperCase(codeDigit);
}
return codeUpperCase;
}

Alex Bratkovskij
5,329 PointsOke, I went back and checked. So thats the working bit except for IAE:
private String normalizeDiscountCode(String discountCode){
if(Character.isLetter(discountCode.charAt(0)) || discountCode.charAt(0) == '$'){
this.discountCode = discountCode.toUpperCase();
} else {
System.out.println("SMTH WRONG!");
}
return this.discountCode;
}
basically your code doesnt see which character it needs to pick up:
There are neet commands for String: someString.charAt(i) where i is index(has to be integer value).
Cuz char is normally primitive data type u cant do much with it. What u want is to use wrapper class Character (which happens to be same thing) and use method within this object called isLetter(ch) where u use primitive char, it returns a boolean (true/false). so it looks like this: Character.isLetter(ch)
about primitives and wrapper classess: https://www.tutorialspoint.com/java/java_characters.htm
Hope this was helpfull :)
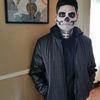
Jorge Otero
16,380 PointsSorry this should be a question for Validating and Normalizing User Input, not for Delivering the MVP. The task is: In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.

Alex Bratkovskij
5,329 PointsAh sorry bud, msired u and then misred the challenge sigh then try this
private String normalizeDiscountCode(String discountCode) {
for( char letter : discountCode.toCharArray()) {
if(! (Character.isLetter(letter) || letter == '$')) {
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
I didnt see that we needed to get through all characters :))