Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial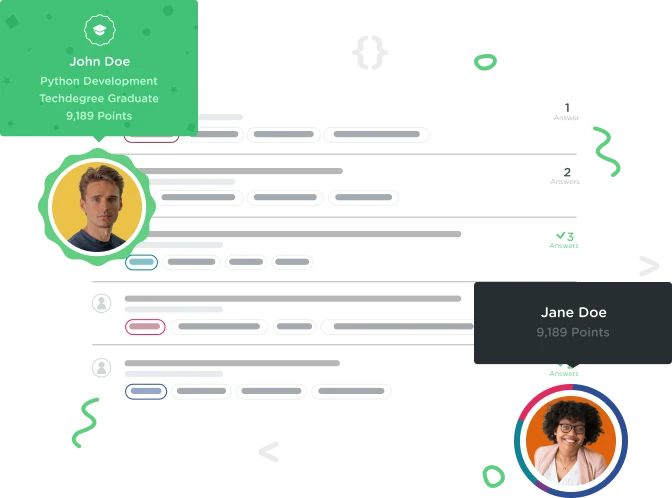

Austin Bynum
1,741 Pointswhat do i need to pass this challenge? there are no compiler errors and i've completed the requested tasks
there are 3 files used in this challenge. per the instructions, i am only supposed to edit CallCenter.java file.
when i run my code, there are no compiler errors and it does everything the steps (in the comments) ask me to do.
the error it gives is "Bummer! Did you remember to call the assist method on the CustomerSupportRep?" and as you can see below...i did call that method. i even did some testing to make sure i completed the third and final step by checking to make sure the object was placed back into the queue...and it is being added back.
what am i missing?
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
do {
playHoldMusic();
} while (mSupportReps.poll() == null);
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
if (mSupportReps.poll() != null) {
csr = mSupportReps.poll();
csr.assist(customer);
mSupportReps.add(csr);
}
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
2 Answers
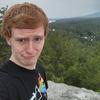
Brendon Butler
4,254 PointsSince the "poll()" method returns a CustomerSupportRep instance you need to have that instance be saved in a variable, then use the assist method off of that particular instance. So instead of using while (mSupportReps.poll() == null);
you'd want to use while ((csr = mSupportReps.poll()) == null);
. That way you're saving the variable and checking for null.
Now that you have your variable you'll want to make sure that you don't coll that "poll()" method again or else it will return a different instance. So remove your second null check and the line where you assign the csr (inside your second null check). Then run your code and it should run fine.
Except for one issue. If there is a rep available before you get to the loop. Since you have a "do-while" loop, it will play the waiting music even if there is a rep available. To fix this. Change your "do-while" loop to just a "while" loop.
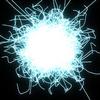
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Austin,
The main trouble with this is that you'e using a do while loop, which is always going to run at least once, so you might end up playing the music while a customer is on hold, and risking customer objects getting stuck in the que, I'd recommend just a regular while loop.
Secondly you did exactly what I did when I first did this, and you used poll() turns out poll is automatically going to grab something so, no matter if it's there to grab or not, so this will run into trouble with your null checks. peek() is probably the method you want, it just looks and sees without acting on the que if something is available, which can lead to unintended bugs, so just fix the do while to just be a while, and change the poll() to peek() and you should be good.
Thanks, hope this helps.
Austin Bynum
1,741 PointsAustin Bynum
1,741 Pointsi see the light now!!! thanks. i now remember in class him showing us how to compare a value and assign it to a variable at the same time just like that. the code smelled bad on that second null check...i should have thought on that more. oh well, thanks again!