Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial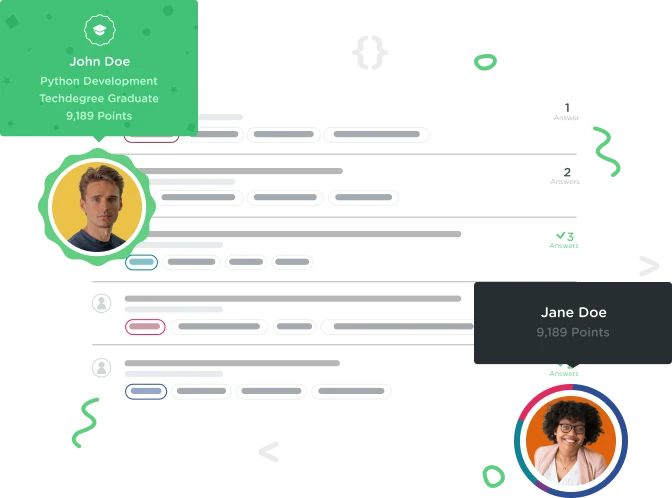
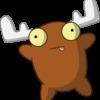
Jon Helmus
7,312 PointsWhat am i getting wrong here?
yet again, another problem i am having an issue understanding.
class Student:
name = "Jon"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if self.grade => 50:
return praise
return reassurance
4 Answers

Unsubscribed User
6,415 PointsVishal's is correct, but it isn't efficient. It's simpler to call the actual methods that achieve these same results. You use "self." with the praise() and reassurance() methods since they're called on the instance of "Student."
Also, just a sidenote, your operators for "greater than or equal to" are reversed and if you ran that, you'd get a syntax error. Greater than or equal is signified by ">=" and likewise with "less than or equal to" being "<=."
Here's the code:
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
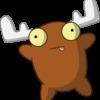
Jon Helmus
7,312 PointsThank you everyone. I'm shaking the spiders outta my brain and getting back into the swing of python things.
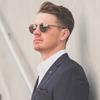
Cameron Sprague
11,272 PointsI was struggling with this concept too. This worked for me. Ask me any questions about the code below and I will see if I can help.
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
else:
return self.reassurance()

Kevin Koch
Full Stack JavaScript Techdegree Student 3,767 PointsI think you're the only one that get the instructions right non of the above use and else and you're the underrated comment

Vishal Gali
5,761 PointsYour issue is just some minor misunderstanding first the question explicitly says result of praise and reassurance not he actual method second grade is not an attribute for self, it is a separate argument. the question said if grade is below or above 50, which means you only need to put grade in.
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return "You inspire me, {}".format(self.name)
return "Chin up, {}. You'll get it next time!".format(self.name)

Khem Myrick
Python Web Development Techdegree Graduate 18,705 PointsThank you! I was getting caught up trying to make grade an attribute of student. When it told me grade wasn't an attribute of student, I assumed I was supposed to make it into an attribute, rather than having it be its own argument. (Calling the praise() and reassurance() methods worked just fine, when I stopped insisting on checking self.grade rather than just grade).

Sanjay Singh
Courses Plus Student 168 PointsI add my one-liner code if someone prefers:
class Student:
name = "John"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
return self.praise() if grade > 50 else self.reassurance()

mourad marzouk
5,560 Pointswhy does it have to be self.praise or self.reassurance?
Jon Helmus
7,312 PointsJon Helmus
7,312 PointsAlright, I need you make a new method named feedback. It should take an argument named grade. Methods take arguments just like functions do. You'll still need self in there, though. If grade is above 50, return the result of the praise method. If it's 50 or below, return the reassurance method's result.