Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial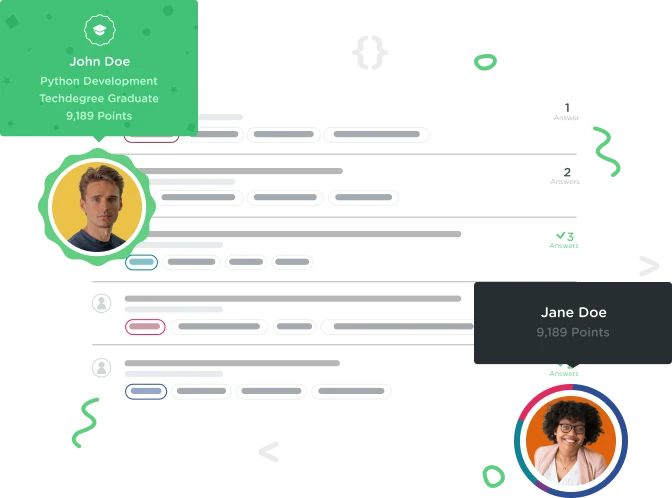

Remy Benza
6,259 PointsValidating user input with Java.util.Scanner;
I've been building a Hotel Reservation System application. It's console based and enables the user to book different type of rooms at the Hilton Hotel in New York.
You can find the application and all the code here:
https://github.com/rbenza/HotelReservationSystem
The programs runs fine when the user provides the right input ('E', 'B' or 'S' for roomtypes Economy, Business or Suite). But I want my app to keep reprompting the user when he provides the wrong input (like a number or different character).
I tried this with an if/else statement and also with a do/while loop but it does not work the way it should. See the code below.
Any suggestions?
Public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to Hilton New York!" + "\n");
System.out.printf("The price of an Economy room is %d US dollars per night. There are %d available for reservation.%n", hiltonNY.getPriceEconomyRoom(), hiltonNY.getEconomyRooms());
System.out.println("The price of an Business room is " + hiltonNY.getPriceBusinessRoom() + " US dollars per night." + " There are " + hiltonNY.getBusinessRooms() + " available for reservation.");
System.out.println("The price of a Suit is " + hiltonNY.getPriceSuit() + " US dollars per night." + " There are " + hiltonNY.getSuits() + " available for reservation.");
System.out.printf("%nWhich roomtype do you want to book? Choose 'E' for Economy, 'B' for Business or 'S' for Suit.%n");
String roomType = scanner.next().toUpperCase();
if (!roomType.matches("[EBS]")) {
System.out.println("Wrong input. Please provide an 'E', 'B' or 'S'");
scanner.next().toUpperCase();
} else
System.out.printf("%nHow many rooms do you want to book?%n");
int numberOfRooms = scanner.nextInt();
if (numberOfRooms < 0 || numberOfRooms > 61) {
System.out.println("Wrong input. Please provide the number of room(s) you want to book.");
scanner.nextInt();
} else
switch (roomType) {
case "E":
new Reservations().bookEconomyRoom(numberOfRooms);
System.out.printf("Booked %d Economy room(s). There are %d Economy room(s) left for reservation.", numberOfRooms, hiltonNY.getEconomyRooms());
break;
case "B":
new Reservations().bookBusinessRoom(numberOfRooms);
System.out.printf("Booked %d Business room(s). There are %d Business room(s) left for reservation.", numberOfRooms, hiltonNY.getBusinessRooms());
break;
case "S":
new Reservations().bookSuit(numberOfRooms);
System.out.printf("Booked %d Suit(s). There are %d Suit(s) left for reservation.", numberOfRooms, hiltonNY.getSuits());
break;
}
}
}
2 Answers
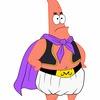
<noob />
17,062 PointsYou can use a while loop or a do while loop which will keep prompt the user as long as he provide wrong input and u can use a try catch and catch exceptions

Remy Benza
6,259 PointsCan you provide the code?