Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial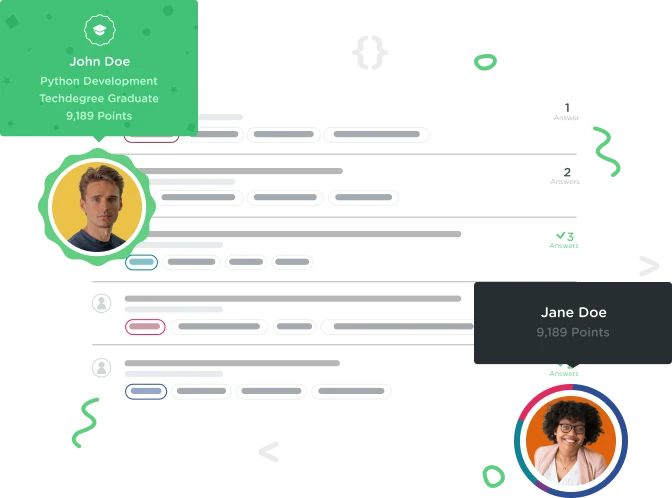

Daniel Silva
5,353 PointsValidating User Input Java
I have a simple command line program that calculates wage and overtime wages.
Everything from the scanner works except the if statement to check if the user's choice is yes or no. Even if the user types yes or no the program runs the last else statement and ends the program. Any help is appreciated.
import java.util.Scanner;
public class WageCalculatorApp {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner input = new Scanner(System.in);
System.out.print("Base Rate: ");
double baseRate = input.nextDouble();
System.out.print("Hours: ");
int hours = input.nextInt();
double overtimeMultiplier;
System.out.print("Would you like to enter an overtime multiplier (yes/no)?");
String choice = input.nextLine();
input.nextLine();
if (choice.equalsIgnoreCase("no")) {
WageCalculator WC = new WageCalculator(baseRate, hours);
System.out.printf("Base wage: %.1f%nOvertime Rate: %.1f%nTotal Wage: %.1f",
WC.baseWage(), WC.overtimeWage(), WC.totalWage());
} else if (choice.equalsIgnoreCase("yes")) {
overtimeMultiplier = input.nextDouble();
WageCalculator WC1 = new WageCalculator(baseRate, overtimeMultiplier, hours);
System.out.printf("Base wage: %.1f%nOvertime Rate: %.1f%nTotal Wage: %.1f",
WC1.baseWage(), WC1.overtimeWage(), WC1.totalWage());
} else {
System.out.println("Choice Not Valid");
}
}
}
1 Answer
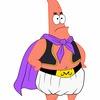
<noob />
17,062 PointsFrom a look of it ur formatting isnβt good in the system.out.printf, what is if%? u canβt use if statement in print commands
Daniel Silva
5,353 PointsDaniel Silva
5,353 PointsI actually figured it out. Also, that isn't if%. If you look closely, it's a 1 as in the number one. You can do this do display float and double values a certain length after the decimal point.