Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial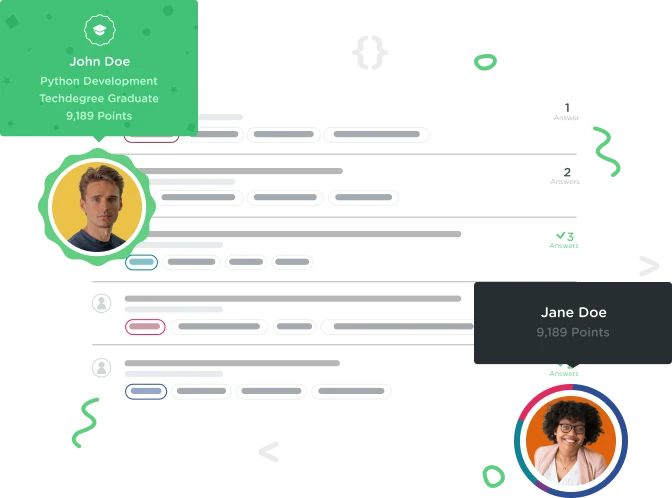

Mamta Chaudhary
527 PointsUsing multiple Classes.
How Would I use the guestName, guestAddress, and others in the other public class Main()? When I use name = input.nextLine();, it states that it "cannot resolve symbol "name"". I want to use the private variables from the Identification class and implement them in the Main class.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Identification guest = new Identification();
guest.Identification();
System.out.println("Welcome to the Motel");
System.out.println("Please answer the following Questions to Check In: ");
System.out.println("Enter your Full Name: ");
name = input.nextLine();
System.out.println("Enter your Address: ");
address = input.nextLine();
System.out.println("Date of Birth: ");
dateOfBirth = input.nextInt();
System.out.println("Enter your Identification Card Number or SSC number: ");
iD = input.nextInt();
}
}
public class Identification {
private String guestName;
private String guestAddress;
private String guestSignature;
private int guestDateOfBirth;
private int guestIdentificationNumber;
public Identification(String guestName, String guestAddress, String guestSignature, int guestDateOfBirth, int guestIdentificationNumber) {
this.guestName = guestName;
this.guestAddress = guestAddress;
this.guestSignature = guestSignature;
this.guestDateOfBirth = guestDateOfBirth;
this.guestIdentificationNumber = guestIdentificationNumber;
}
public String getGuestName () {
return guestName;
}
public void setGuestName (String name){
guestName = name;
}
public String getGuestAddress () {
return guestAddress;
}
public void setGuestAddress (String address){
guestAddress = address;
}
public String getGuestSignature () {
return guestSignature;
}
public void setGuestSignature (String signature){
guestSignature = signature;
}
public int getGuestDateOfBirth () {
return guestDateOfBirth;
}
public void setGuestDateOfBirth ( int dateOfBirth){
guestDateOfBirth = dateOfBirth;
}
public int getGuestIdentificationNumber () {
return guestIdentificationNumber;
}
public void setGuestIdentificationNumber ( int iD){
guestIdentificationNumber = iD;
}
}
1 Answer
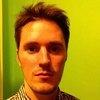
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsThe variable name
hasn't been declared yet. Try adding String
in front of it, to declare the variable instead of just referencing it:
String name = input.nextLine();
You can set the property on your guest
instance with the setter method:
guest.setGuestName(name);
The name
property of Identification
is private, but you can set it with the public method setGuestName
.