Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial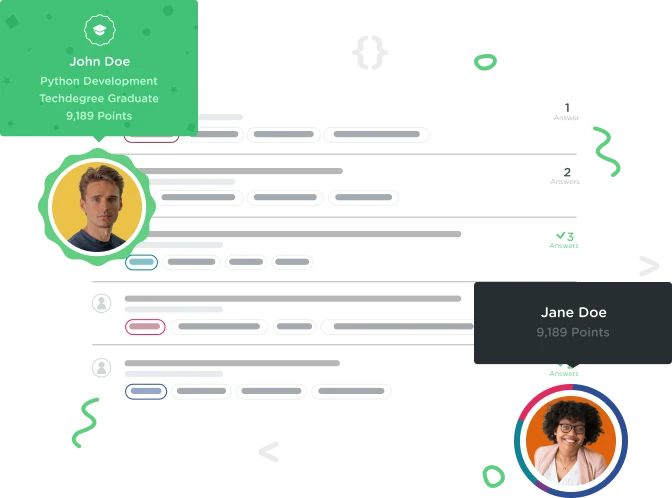
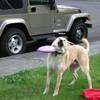
Maxwell Nelson
9,465 PointsUsing classes/interfaces as member variables and method parameters.
I am on working on the Karaoke Machine project on the Java Track. Most everything makes sense to me, but connecting the dots here seems to be a tad more difficult.
So for example:
package com.teamtreehouse.model;
import java.util.List;
import java.util.ArrayList;
public class SongBook {
private List<Song> mSongs;
public SongBook() {
mSongs = new ArrayList<Song>();
}
public void addSong(Song song) {
mSongs.add(song);
}
public int getSongCount() {
return mSongs.size();
}
}
This is the SongBook class. Under the class declaration there is -- private List<Song> mSongs; -- What is the reason behind using a list interface, or any interface for that matter in a member variable? Secondly, I am struggling to grasp member fields that contain classes.
For example:
package com.teamtreehouse;
import com.teamtreehouse.model.SongBook;
public class KaraokeMachine {
private SongBook mSongBook;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
}
}
This is the KaraokeMachine class. Under the class declaration there is -- private SongBook mSongBook; -- why is this? I suppose my question is this: Can someone explain the logic behind using an interface and/or a class in a member variable. I've watched the videos and looked online over and over buy the complexity of wordage makes the reasoning elusive to me.
Thanks so much!
2 Answers
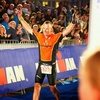
Steve Hunter
57,712 PointsHi Maxwell,
The list interface allows you to use all the methods that the interface implements. This makes them more flexible than an array, for example. So the line,
private List<Song> mSongs;
Defines that the member variable mSongs
will hold a list of objects that are all instances of the Song
class.
Similarly,
private SongBook mSongBook;
This line says that the member variable mSongBook
will hold an instance of the class SongBook
. You've said that having trouble understanding member variables that contain classes. They all do! Take, for example:
private String mFullName;
private int ageOfUser;
private Date dateOfBirth;
These more familiar data types may sit more easily with you but they are just classes; implementations which include their own methods and inherit from classes above. The self-created classes like SongBook
and Song
work in a similar way. Such that, as you would expect, an instance of SongBook
, like mSongBook
, will contain many instances of Song
, i.e a list of Song
instances held in mSongs
, the list; the individual instance of Song
called song
is passed into the method addSong(Song song)
. Let's try a real-world example. Such that an instance of Car
could contain many instances of Seat
or Wheel
.
I hope that helps a little. If not, just shout and I'll have another go!
Steve.
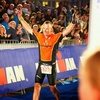
Steve Hunter
57,712 PointsAlso, have a read through this post which has a dig around in classes, instances and objects etc. That may help a little too.
Steve.
Maxwell Nelson
9,465 PointsMaxwell Nelson
9,465 PointsOkay it is starting to make sense to me. Thank you for the quick response! A follow up question though: You're saying -- private List mSongs; -- holds a list of of all the objects of Song, this includes its methods as well, correct? Also, whats the point of storing all the objects of an instance in a list like this, vs just having the list in song and adding -- private Song mSong as this would also give this class access to instances of Song -- is this just a "personal coding style" kind of thing or is it required for compatibility sake?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Maxwell,
Yes, the
List
of typeSong
holds a list ofSong
instances/objects. And, yes, each of those instances hold all of the methods that are available from theSong
class and any methods inherited from superclasses. Further than this, though, theList
has its own methods that make it easier to manipulate the collection ofSong
, or any other, objects. Lists have functionality themselves, irrespective of what type of instance the list holds. Make sense?In this current implementation, which is created largely to work through collections as a principle rather than to be the best way to implement a karaoke machine, is using a list of available songs for the user to select from and sing. For this, all the songs need to be in one accessible place (the
SongBook
) and then we need another collection ofSong
objects that are the list ofSong
objects yet to be performed/murdered. That's getting ahead a bit, perhaps. I'm not sure how you are envisaging "just having the list in song and adding -- private Song mSong" - I'm not clear on that so am struggling to find an answer for that part. Can you explain what you mean and I'll get back to you.I don't think there are many style decisions in this code segment. While there are decisions being made to demonstrate the learning content being covered, i.e. how to use data collections, the choices are well made and relevant. Yes, there are certainly better (and more complicated) methods of doing this with databases and such like, the List example is valid for a console program like this.
Get back to me on the on the
Song
thing, above, and I'll try to explain.Steve.
Maxwell Nelson
9,465 PointsMaxwell Nelson
9,465 PointsOkay that all makes complete sense - thank you so much! Sorry for the muddled question, I believe you answered that question indirectly but let me try and explain it further. In order for one class to be able to implement another class's methods, one could simply put (Assuming the a list was created within the Song class)
public class SongBook { private Song mSongs; }
So why would it be:
public class SongBook { private List < Song > mSongs; }
I believe from what you were saying the reason is because this specific lesson is largely targeted at understanding iterating through collections, not necessarily what may or may not be the most efficient way of creating this karaoke machine. However, if this has purpose i.e. "it must be private List<Song> mSongs; due to '______' " please let me know.