Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial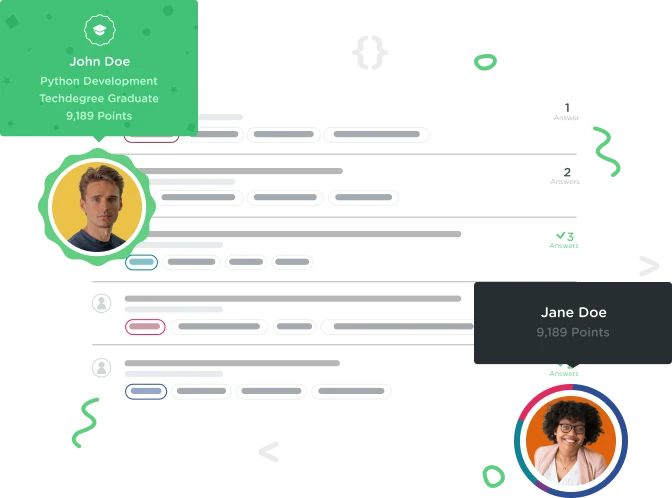

Sahil Kapoor
8,932 PointsUse of Arrow function in objects?
function particle(){
this.x = 100;
this.show = () => {
console.log(this.x);
}
}
let p = new particle();
let showX = p.show;
console.log(showX); // output - 100
Here arrow functions helped binding the instance p with the function show that's why it shows the same result even after being referenced to a variable. But MDN(Mozilla Developers network) have written, "arrow function expressions are best suited for non-method functions". But in this example we saw that it helped us from making an error by binding instance with the function. Any help regarding arrow function and its use would be really great..
1 Answer
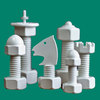
Steven Parker
231,007 PointsIf you are sure you know what you're doing, you can disregard that "best suited" suggestion. But they probably say that because the value is now tied to directly to the object and not the method context, and the resulting behavior may be unexpected and confusing:
let p = new particle();
let showX = p.show;
let n = new particle();
let showN = n.show;
n.x = 99;
var x = 222;
// Function Type: Normal Arrow
p.show(); // 100 100
showX(); // 222 100
showX.call(n); // 99 100
showN.call(p); // 100 99
With the arrow function, the value produced when called explicitly with a different context is the opposite of what you'd get if the function had a conventional definition.
Sahil Kapoor
8,932 PointsSahil Kapoor
8,932 PointsSo in arrow function the show method in bind to the instance created (like p) and not to "this."? What's why showX.call(n) shows the value of p.x and not n.x ??
Steven Parker
231,007 PointsSteven Parker
231,007 PointsIn a conventional function, "this" refers to the context that the call is performed in, so
p.show()
gives the value ofp.x
,showX()
gives the value of the global "x" andshowX.call(n)
gives n.x. But arrow functions don't bind "this" so the reference to "this" becomes the object where the definition is.Sahil Kapoor
8,932 PointsSahil Kapoor
8,932 PointsYa now I get the idea thanks a lot for the help.