Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial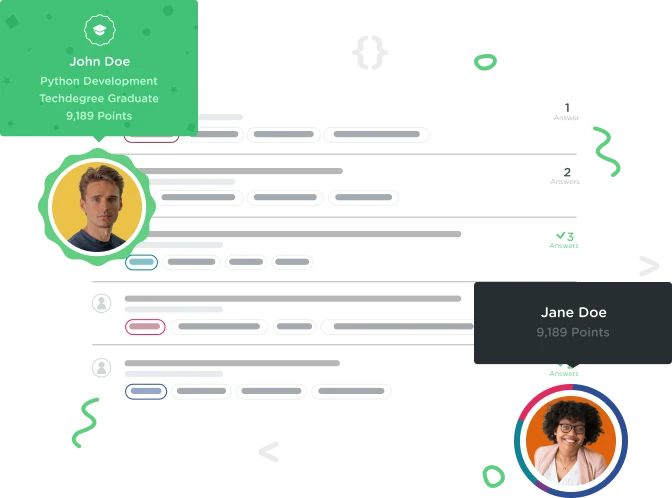
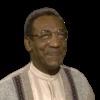
Jake Salo
13,175 PointsUnity5 - orbiting mouse controlled camera goes through terrain
i have a custom roll-a-ball game with a mouse orbiting camera and im having serious issues with it. basically, whenever the player nears something with a collider (i.e. a wall or a tree or rock etc...), then the camera inverts it's coords and either goes outside of the map or inside of the player. I have looked at the following thread and tried that solution but couldn't get that working so maybe someone on here could help me out, thanks.
http://answers.unity3d.com/questions/750104/orbiting-mouse-controlled-camera-goes-thru-terrain.html
using UnityEngine;
using System.Collections;
public class CameraController : MonoBehaviour
{
public GameObject player;
private Camera camera;
public Transform target;
public float distance = 10.0f;
public float xSpeed = 120.0f;
public float ySpeed = 120.0f;
public float yMinLimit = 20.0f;
public float yMaxLimit = 150.0f;
public float distanceMin = 5.0f;
public float distanceMax = 15f;
private Rigidbody rigidbody;
float x = 0.0f;
float y = 0.0f;
private Vector3 offset;
// Use this for initialization
void Start()
{
Vector3 angles = transform.eulerAngles;
x = angles.y;
y = angles.x;
camera = GetComponent<Camera>();
rigidbody = GetComponent<Rigidbody>();
// Make the rigid body not change rotation
if (rigidbody != null)
{
rigidbody.freezeRotation = true;
}
Vector3 v = new Vector3(0.0f, 0.0f, 5);
offset = transform.position - (player.transform.position - v);
}
// Update is called once per frame
void LateUpdate()
{
Debug.Log(camera.transform.position);
transform.position = player.transform.position + offset;
if (target)
{
x += Input.GetAxis("Mouse X") * xSpeed * distance * 0.02f;
y -= Input.GetAxis("Mouse Y") * ySpeed * 0.02f;
y = ClampAngle(y, yMinLimit, yMaxLimit);
Quaternion rotation = Quaternion.Euler(y, x, 0);
distance = Mathf.Clamp(distance - Input.GetAxis("Mouse ScrollWheel") * 5, distanceMin, distanceMax);
RaycastHit hit;
if (Physics.Linecast(target.position, transform.position, out hit))
{
distance -= hit.distance;
}
Vector3 negDistance = new Vector3(0.0f, 0.0f, -distance);
Vector3 position = rotation * negDistance + target.position;
transform.rotation = rotation;
transform.position = position;
}
}
public static float ClampAngle(float angle, float min, float max)
{
if (angle < -360F)
angle += 360F;
if (angle > 360F)
angle -= 360F;
return Mathf.Clamp(angle, min, max);
}
}
Alan MattanΓ³
Courses Plus Student 12,188 PointsAlan MattanΓ³
Courses Plus Student 12,188 PointsProbably this has to be in game development.