Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial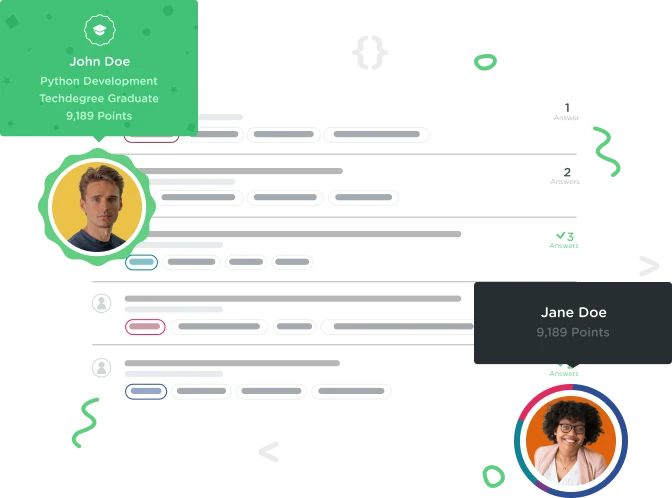
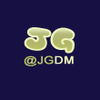
Jonathan Grieve
Treehouse Moderator 91,254 PointsUnderstanding Type Casting in Java
Hi all.
Here's some notes I've done about Type casting. It's based on examples from the video and the Oracle docs. This is where it starts to get tricky for me to get my head around but hopefully this is not too far away from what I need to know. :)
Typecasting
In Java every type of Class is an extension of the type Object. Inheritance allows you to use an instance anywhere one of its superclasses is required.
It's possible for a parent class to have another type and explicitly cast itself as a child (or subclass). I believe that is called Typecasting.
Downcast - going down the inheritance chain of objects
Upcasting is always possible but downcasting can cause runtime exceptions if a casted object is not known as available to Object.
We can use instanceof keyword to make a compile time check that Object is correctly casted ???
Thought: Typecasting is assignment of an object type to an existing object type so it then switches to the new type?
Example of Casting.
//a new instance of an object with values passed in.
Treet treet = new Treet("craigdennis", "Example text", new Date());
Upcasting Example:
Object obj = treet;
Downcasting Example
Object variableName = (NewObjectTypecast) obj where obj is a reference to the main Object.
- Best practice to use instanceOf() before performing a downcast
A runtime check that an object can be cast to a particular type might look like this
if (obj instanceof MountainBike) { MountainBike myBike = (MountainBike)obj; }
https://docs.oracle.com/javase/tutorial/java/IandI/subclasses.html
Summary:
Casting shows the use of an object of one type in place of another type.
e.g.
Object obj = new MyObject(); an upcast. an implicit cast. showing an object as both an Object and a MyObject
If we write a downcast e.g. MyObject myobj = obj; the compiler doesn't know about MyObject. Using Explict casting.. or downcasting we show the compiler our intention to create that class and to treat it as a particular type.
instanceof
is the keyword that checks that the cast you're trying to make on an object is correct and you can cast it as that type.
Lakshmi Narayana Dasari
1,185 PointsLakshmi Narayana Dasari
1,185 PointsI am bit confused with the ise of instanceof. For example:
Mountain m = new Mountain(); Object obj; if(obj instanceof Mountain) // here obj is not an instanceof mountain but how does this block executes. { Mountain mou = (Mountain)obj; }