Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial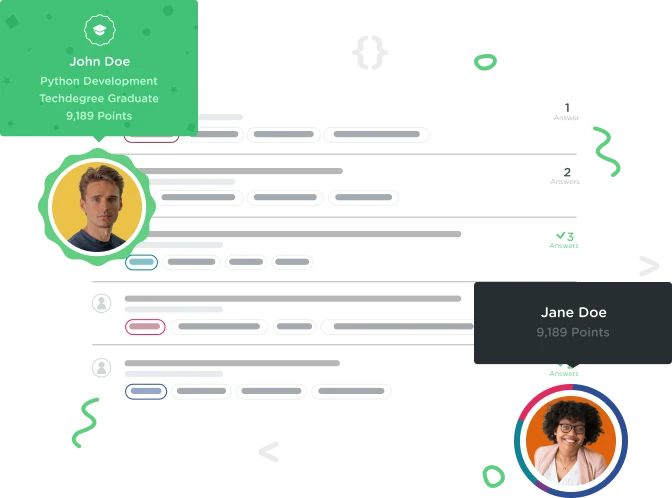
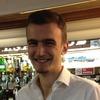
Stefan Novak
6,791 PointsUnable to create requested service
Hello everyone.
This is a tough one, I've been at it for about an hour now and can't fix it myself, so I'm asking for some help.
I've got a feeling the issue is to do with outdated dependencies, the problem is when trying to update it myself I get so confused in all the terminology and jargon, and don't know what things I need to import. The whole dependency management concept still confuses me.
When I run this program I get a huge error, but I think I've isolated it to this 'Unable to create requested service' issue, maybe something to do with connecting to the H2 database?
Anyway, here is my code, I really hope I can get this working because so far, this hibernate course has been great and I'm really enjoying it.
plugins {
id 'java'
}
group 'com.teamtreehouse'
version '1.0-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
// compile 'org.hibernate:hibernate-core:5.1.0.Final' OLD
compile 'org.hibernate:hibernate-core:5.4.2.Final' //current
// compile 'com.h2database:h2:1.4.191' OLD
testCompile group: 'com.h2database', name: 'h2', version: '1.4.199' //current
compile 'javax.transaction:jta:1.1'
// https://mvnrepository.com/artifact/org.hibernate/hibernate-core
// compile group: 'org.hibernate', name: 'hibernate-core', version: '6.0.0.Alpha2', ext: 'pom'
// testCompile group: 'com.h2database', name: 'h2', version: '1.4.199'
// compile group: 'javax.transaction', name: 'jta', version: '1.1'
}
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="connection.driver_class">org.h2.Driver</property>
<property name="connection.url">jdbc:h2./data/contactmgr</property>
<!-- SQL dialect -->
<property name="dialect">org.hibernate.dialect.H2Dialect</property>
<!-- Create the database schema on startup -->
<property name="hdm2ddl.auto">create</property>
<!-- Show the queries prepared by Hibernate -->
<property name="show_sql">true</property>
<!-- Names the annotated entity classes -->
<mapping class="com.teamtreehouse.contactmgr.model.Contact"/>
</session-factory>
</hibernate-configuration>
package com.teamtreehouse.contactmgr;
import com.teamtreehouse.contactmgr.model.Contact;
import com.teamtreehouse.contactmgr.model.Contact.ContactBuilder;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.service.ServiceRegistry;
public class Application {
//Hold a reusable reference to a SessionFactory (since we only need one)
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
//Create a StandardServiceRegistry
final ServiceRegistry registry = new StandardServiceRegistryBuilder().configure().build();
return new MetadataSources(registry).buildMetadata().buildSessionFactory();
}
public static void main(String[] args) {
Contact contact = new ContactBuilder("Stefan","Novak")
.withEmail("stefannovak96@gmail.com")
.withPhone(7735556666L)
.build();
//Open a session
Session session = sessionFactory.openSession();
//begin transaction
session.beginTransaction();
//use the session to save the contact
session.save(contact);
//commit the transaction
session.getTransaction().commit();;
//close the session
session.close();
}
}
package com.teamtreehouse.contactmgr.model;
import javax.persistence.*;
@Entity
public class Contact {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
@Column
private String firstName;
@Column
private String lastName;
@Column
private String email;
@Column
private Long phone;
//Default constuctor for JPA
public Contact(){}
public Contact(ContactBuilder builder) {
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.email = builder.email;
this.phone = builder.phone;
}
@Override
public String toString() {
return "Contact{" +
"id=" + id +
", firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
", email='" + email + '\'' +
", phone=" + phone +
'}';
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Long getPhone() {
return phone;
}
public void setPhone(Long phone) {
this.phone = phone;
}
public static class ContactBuilder {
private String firstName;
private String lastName;
private String email;
private Long phone;
public ContactBuilder(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
//because its not in the constructor...
//we can choose to use this when building a row
public ContactBuilder withEmail(String email) {
this.email = email;
return this;
}
public ContactBuilder withPhone(Long phone) {
this.phone = phone;
return this;
}
public Contact build() {
return new Contact(this);
}
}
}
THANKS <3
1 Answer

khaled adel
3,252 Points<property name="hdm2ddl.auto">create</property>
i beleive it should be "b" instead "d"
<property name="hbm2ddl.auto">create</property>
Stefan Novak
6,791 PointsStefan Novak
6,791 PointsWow eagle eyes! Thank you! Unfortunately a problem still persists lol, but nevermind, I'm going to try and move onto the Spring with Hibernate course and hopefully some issue's will resolve themselves :)
THANK YOU