Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial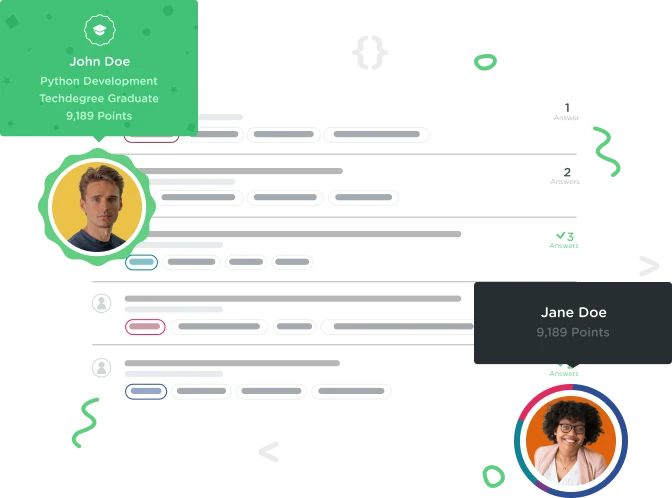

edmond habimana
Courses Plus Student 8,352 PointsTrying to rewrite the Hangman code now that i am done with the course, but i am getting an error.
Can someone please explain to me what the error means and what i did wrong. This is the error:
Exception in thread "main" java.lang.NullPointerException
at com.company.Prompter.inputGuess(Prompter.java:30)
at com.company.Main.main(Main.java:10)
This is the code in the main class
package com.company;
public class Main {
public static void main(String[] args) {
Game game = new Game("treehouse");
Prompter prompter = new Prompter();
prompter.inputGuess();
}
}
This is the code in the Prompter class
package com.company;
import java.util.Scanner;
/**
* Created by edmon on 1/30/2017.
*/
public class Prompter {
public static Scanner scanner = new Scanner(System.in);
private String name;
private Game game;
public Prompter(){
}
public String getName(){
return this.name;
}
public void inputGuess(){
System.out.println("what is your name? ");
this.name = scanner.nextLine();
System.out.printf("%s, what is the word? \n", name);
System.out.println("Please enter a letter to guess. ");
String guess = scanner.nextLine();
game.applyGuess(guess);
System.out.printf("This is your progress so far: ",game.progress());
}
}
And this is the code in the Game class
package com.company;
/**
* Created by edmon on 1/30/2017.
*/
public class Game {
private String answer;
private String hits;
private String misses;
private Prompter prompter;
public Game(String answer){
this.answer = answer.toLowerCase();
hits = "";
misses = "";
}
public void applyGuess(String letter){
if(letter.length() == 0){
throw new IllegalArgumentException( prompter.getName() + " nothing was entered.");
}else{
applyGuess(letter.charAt(0));
}
}
public char normalize(char letter){
if(!Character.isLetter(letter)){
throw new IllegalArgumentException( prompter.getName() + " please enter a letter.");
}else{
letter = Character.toLowerCase(letter);
return letter;
}
}
public void applyGuess(char letter){
letter = normalize(letter);
if(answer.indexOf(letter) != -1){
hits += letter;
}else{
misses += letter;
}
}
public String progress(){
String progress = "";
for(char letters: answer.toCharArray()){
String display = "-";
if(hits.indexOf(letters) != -1){
display += letters;
}
progress += display;
}
return progress;
}
}
Thanks for the help.
2 Answers
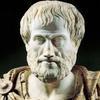
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsHi Edmond,
I only took a quick look over your code and it seems that the "game" field in the Prompter class is never being initialized. In the inputGuess() method you are calling game.applyGuess() without "game" having been initialized, therefore being null and therefore throwing the NullPointerException.
You may want to edit the Prompter constructor to take a Game as an argument.
public Prompter(Game game){
this.game = game;
}
and in your Main class
public static void main(String[] args) {
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
prompter.inputGuess();
}
Kind Regards, Florian

edmond habimana
Courses Plus Student 8,352 PointsThanks for the help Florian!!