Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial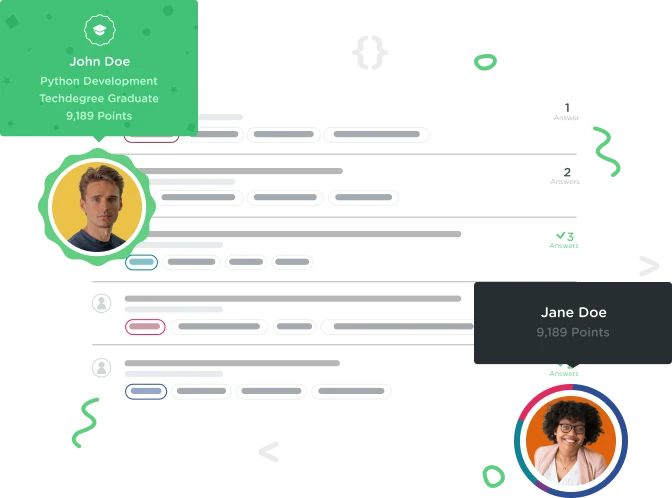

Jerad Millay
14,396 PointsTrouble adding class to element sibling?
I am having trouble coding the challenge of adding the "hightlight" class to the <button> sibling element <p> when <button> is clicked. Obviously, there is something I am missing or incorrectly calling. Please help!
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'button') {
let button = document.querySelectorAll('button');
let Li = button.parentNode;
let p = button.previousElementSibling;
p.addClassName("hightlight");
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
1 Answer

Viktor Doska
2,138 PointsWell, first of all, the task says Add the code to create this behavior on line 5 . Now, let's look at your code:
let button = document.querySelectorAll('button');
let Li = button.parentNode;
You DONT need this 2 lines because your button (the one that is clicked) is e.target And you don't need line with declaring li variable because to find a sibling you dont need to know the parent
let p = button.previousElementSibling;
p.addClassName("hightlight");
And this is pretty much the correct answer, you just need to make it 1 line and remove excess variables:
e.target.previousElementSibling.className = 'highlight';

Jerad Millay
14,396 PointsThank you Viktor. With learning all of this, I tend to complicate the coding. The simple line you provided makes complete sense now. Thanks again for your assistance!
Jerad Millay
14,396 PointsJerad Millay
14,396 PointsMisspelled "highlight", changed in coding above and still receiving same incorrect error...