Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial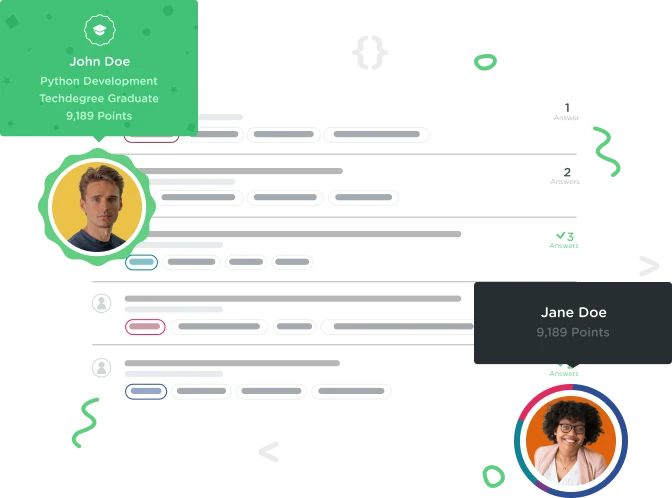

Mark Hess
6,360 PointsTreeStory unfinished - posting again...
Posting my problem again since still have received no feedback on my previous post (no one at TreeHouse watches these forums?). I continue to get an error on the third TODO for not rendering the result story, however the result story renders fine when I rend the tests. I honestly don't see what I could do differently
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
// write your code here
Prompter prompter = new Prompter();
System.out.printf("Make a story! Write a fill in the blank story with an underscore on each side of the variable like __noun/adjective/etc__");
String story = null;
try {
story = prompter.promptForStory();
} catch (IOException ioe) {
ioe.printStackTrace();
}
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
// Implement - Print out the results that were gathered here by rendering the template
String result = tmpl.render(results);
System.out.printf("\nYour TreeStory:%n%n%s", result);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return list of words
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
// Implement - Prompt the user for the response to the phrase,
// make sure the word is censored, loop until you get a good response.
System.out.printf("%nEnter your word for phrase: %s%n", phrase);
String response = null;
try {
response = mReader.readLine().toLowerCase().trim();
} catch (IOException ioe) {
ioe.printStackTrace();
System.exit(0);
}
while(mCensoredWords.contains(response)) {
System.out.printf("%nThe word you chose is not allowed, choose again: %n");
response = mReader.readLine().toLowerCase().trim();
}
return response;
}
public String promptForStory() throws IOException{
String response = null;
System.out.print("\nEnter your story: ");
try {
response = mReader.readLine();
} catch (IOException ioe) {
ioe.printStackTrace();
System.exit(0);
}
return response;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
2 Answers
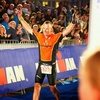
Steve Hunter
57,712 PointsHi Mark,
Sorry, I didn't see your earlier thread - I must have missed it.
Without going through all your code I can see one little difference to my code (not that my code is the holy grail!!). In Main
you've correctly called prompter.run(tmpl)
. And in that method, you've output the story.
My attempt at this had the run
method return a string and I output that String from Main
.
I uploaded my code into Github, if that'll help. Have a look through it, if you like.
What error do you get in the challenge compiler? That may articulate what's not fitting the bill.
Steve.

Seth Kroger
56,416 PointsI see you are always converting the words to lowercase, but that isn't stated in the requirements. Can you try your code without using toLowerCase() and see if it will pass?

Mark Hess
6,360 PointsThats interesting idea except on the comparison, wouldn't it be wise to not trust the user? I utilized Steve's code directly and was able to pass the grader. I think its somewhat upsetting since what my code returned is actually correct.

Seth Kroger
56,416 PointsYou could use mCensoredWords.contains(response.toLowerCase()) to make sure input like "DoRk" is caught but not alter the input otherwise.

Mark Hess
6,360 PointsThis is true. Well I'm finally through the exercise now. Bothersome part is I didnt necessarily do anything wrong, it just wasn't exactly as the grader anticipated.
Mark Hess
6,360 PointsMark Hess
6,360 PointsThank you for the reply Steve, let me test now. As for the 'error' I'm getting its stating I didnt finish TODO 3, I'm not presenting the story to the user. Makes no sense since from what I can see I am but for whatever reason the editor on TreeHouse is not.
Just ran your idea and still getting
Looks like you didn't present the TreeStory (3)
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI just found an error in my code! Ha! Pretty normal, really! You'll need to change the censored words output string from
phrase
toanswer
at line 90 ofPrompter
.Your code seems more correct than mine. So I'll keep looking at why it isn't passing.
Mark Hess
6,360 PointsMark Hess
6,360 PointsI changed the
response
toanswer
(not sure how that would do anything, but I don't know how the grader operates).phrase
is the input string for that method though, it wasn't on line 90. Either way made some changes and still getting same incorrect.Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI've not uploaded the final version of that - you've got an initial commit, not the end result. I'll dig out the source and upload the revised stuff.
Mark Hess
6,360 PointsMark Hess
6,360 PointsOh ok, so do need to wait for changes to be made on the backend?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo, that's just my code in Github, not the challenge code. The challenge will be fine.
Mark Hess
6,360 PointsMark Hess
6,360 PointsWell I used your code directly and other than TODO's needing to be removed it worked fine. However the logic and results are still the same. Honestly think there is something the grader is doing or looking for that isn't apparent to us students.