Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial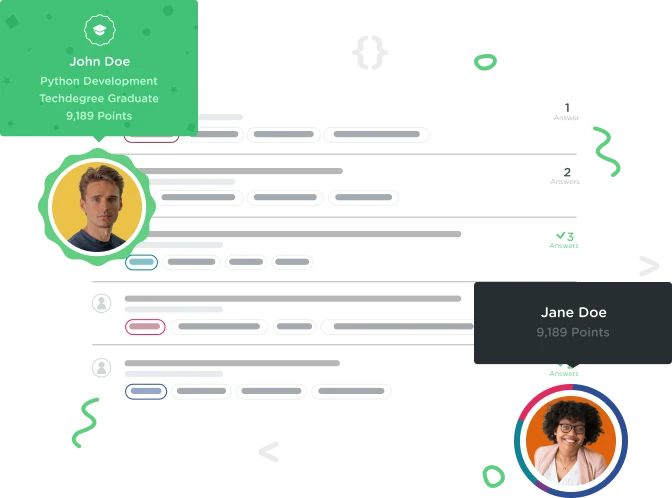
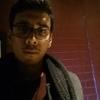
Giovanny Hernandez
Full Stack JavaScript Techdegree Student 8,773 PointsTreehouse story
When I submit my code it tells me that the third test was not pass. It is saying my code never displays but when I run it in my IDE this is the outcome: Please create a story with Format for blanks THe story and goal Please fill in the blank for: story treehouse Please fill in the blank for: goal dweeb Censored word learning Your TreeStory:
THe treehouse and learning Process finished with exit code 0
package com.teamtreehouse;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompt = new Prompter();
Template tmpl = new Template(prompt.promptForStory());
try {
prompt.run(tmpl);
} catch (IOException e) {
e.printStackTrace();
}
}
}
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) throws IOException {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String story = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", story);
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.println("Please fill in the blank for: " + phrase);
String word = mReader.readLine();
// String fWord = "";
for (String censor : mCensoredWords) {
if (word.equalsIgnoreCase(censor)) {
System.out.println("Censored word");
word = mReader.readLine();
}
}
return word;
}
public String promptForStory(){
System.out.println("Please create a story with __Format__ for blanks");
String story = null;
try {
story = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return story;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story

Simon Coates
28,694 PointsPeople have been having a lot of complaints for this challenge. You should be able to find the complete code in an earlier forum post or by searching google for treehouse and some term particular to this challenge. People keep complaining that their code works fine, so it's a matter to tracking some minor thing that treehouse cares about to pass the test.
1 Answer

Seth Kroger
56,415 PointsYour promptForWord() method only checks the input for a bad word and re-asks once, then lets that second word through regardless of it being censored or not. What it needs to do is keep re-asking until you get a word that isn't censored.
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsDid you read the story back to the user?