Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial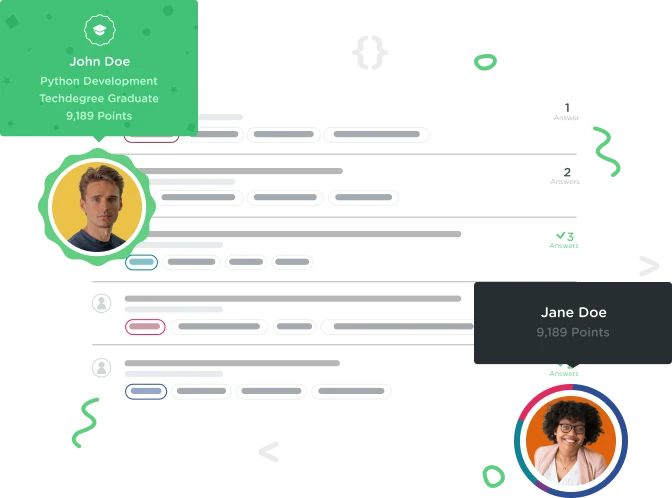

Mikey Ro
6,948 PointsTimer App iOS problem
I am trying to build a simple timer app in Swift. I have a bug where the "seconds" display does not reset to zero after it reaches 60 seconds. instead it continues to count upward. At one minute the timer will look like this "01:60:00" at 1:30 it looks like this "01:90:00" etc. I can't figure out how to fix this. any ideas?
// // ViewController.swift // MemorySportTimer // // Created by mrodin on 2017-12-23. // Copyright © 2017 mrodin. All rights reserved. //
import UIKit import AVFoundation
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
@IBOutlet weak var timerCountdownLabel: UILabel!
@IBAction func timerCountdownSlider(_ sender: UISlider) {
timerCountdownLabel.text = "\(Int(sender.value))"
}
@IBOutlet weak var timerLabel: UILabel!
@IBOutlet weak var tableView: UITableView!
var totalSeconds: Float = 0
var timer = Timer()
var lapTimeArray = [String]()
var audioPlayer = AVAudioPlayer()
var timerIsRunning = false
override func viewDidLoad() {
super.viewDidLoad()
do {
let audioPath = Bundle.main.path(forResource: "Alarm", ofType: ".mp3")
try audioPlayer = AVAudioPlayer(contentsOf: URL(fileURLWithPath: audioPath!))
}
catch {
//ERROR
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Button Functions
//Start Button
@IBAction func startButtonDidTouch(_ sender: Any) {
print("start Tapped")
if !timerIsRunning{
timer = Timer.scheduledTimer(timeInterval: 0.01, target: self, selector: #selector(self.updateTimer), userInfo: nil, repeats: true)
timerIsRunning = true
}
}
//Stop Button Method
@IBAction func stopButtonDidTouch(_ sender: Any) {
if timerIsRunning{
timer.invalidate()
timerIsRunning = false
audioPlayer.stop()
}else{
audioPlayer.stop()
}
}
//Reset button Method
@IBAction func resetButtonDidTouch(_ sender: Any) {
totalSeconds = 0
timerLabel.text = "00:00:00"
lapTimeArray = []
tableView.reloadData()
}
//Time-in button Method
@IBAction func lapButtonDidTouch(_ sender: Any) {
lapTimeArray.append(timerLabel.text!)
tableView.reloadData()
print("lap Tapped")
}
//MARK: Update Timer Method
@objc func updateTimer() {
totalSeconds += 0.01
let totalSecondsTimes100: Int = Int(totalSeconds*100)
let minutes = Int(totalSeconds/60)
let timerChoice = Double(minutes) + 0.1
//formats timer digit sequence
let minStr = (minutes == 0) ? "00" : "0\(minutes)"
let secStr = (totalSeconds < 9) ? "0\(Float(totalSecondsTimes100)/100)" : "\(Float(totalSecondsTimes100)/100)"
switch Int(timerChoice) {
case Int(timerCountdownLabel.text!)!:
timerLabel.text = "\(minStr):\(secStr)"
audioPlayer.play()
timer.invalidate()
timerIsRunning = false
default:
timerLabel.text = "\(minStr):\(secStr)"
}
}
//MARK: - Methods for table view - required
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "lapRecordCell")
cell?.textLabel?.text = lapTimeArray[indexPath.row]
return cell!
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return lapTimeArray.count
}
}
1 Answer
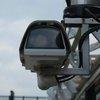
Bruce Röttgers
18,211 PointsSet up a variable for ms, s and min in the update method. Than make if statements. If ms reaches 100 reset it to 0 and add 1 to seconds. Do this for seconds and minutes too.
Simon Di Giovanni
8,429 PointsSimon Di Giovanni
8,429 PointsDoes the “minutes” constant update to 1 once 60 seconds is reached?
So does it count upwards 00:59:00 -> 01:00:00?