Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial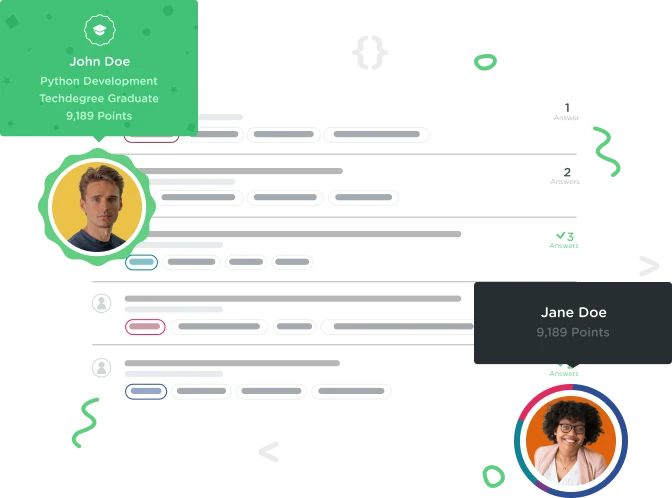

Alex Potham
860 Pointsthis is ultra difficult. please help me
You've seen how random.choice() works. It gets a random member from an iterable (like a list or a string). I want you to try and reproduce it yourself. First, import the random library. Then create a function named random_item that takes a single argument, an iterable. Then use random.randint() to get a random number between 0 and the length of the iterable, minus one. Return the iterable member that's at your random number's index. Check the file for an example.
i know this is all stuff we've covered on its own but combining it all has broken my brain. any help would be appreciated
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
iterable = ["piefaceshowcase"]
def random_item(iterable):
return random.randint
1 Answer
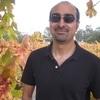
Kourosh Raeen
23,733 PointsAccording to the instruction, the random int value needs to be between zero and one less than the length of the iterable. Also, you need to return the iterable member at that index not the random int. You also, don't need to define and initialize the iterable outside the function:
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
def random_item(iterable):
index = random.randint(0, len(iterable) - 1)
return iterable[index]
Alex Potham
860 PointsAlex Potham
860 Pointsthanks Kourosh for your help.
Levis Vazquez
961 PointsLevis Vazquez
961 Pointsthanks
Shadow Skillz
3,020 PointsShadow Skillz
3,020 PointsHey Kourosh thanks for the help really appreciate it. I have a question i tried to run the same script but just changed the return to index it didn't pass but runs fine on my editor i also have issues with the indenting when doing the challenges when i create a block its four spaces and doesn't pass i cross referenced your script with mine which was the same only differing in spaces and it still wouldn't pass copied and pasted yours pass any ideas if so i would be grateful to hear thanks
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsHi Christian - Your code returning
index
runs fine in your editor but it's not accomplishing what the challenge is asking for so that's why it's not passing. The challenge says: "Return the iterable member that's at your random number's index." which means you need to returniterable[index]
and not justindex
.As for indentation, as long as it is consistent throughout your code then it shouldn't be a problem. I just tried the code I posted above with 2 spaces, 3 spaces, and 4 spaces for indentation and it passed each time.
Hope this helps.